Data Types
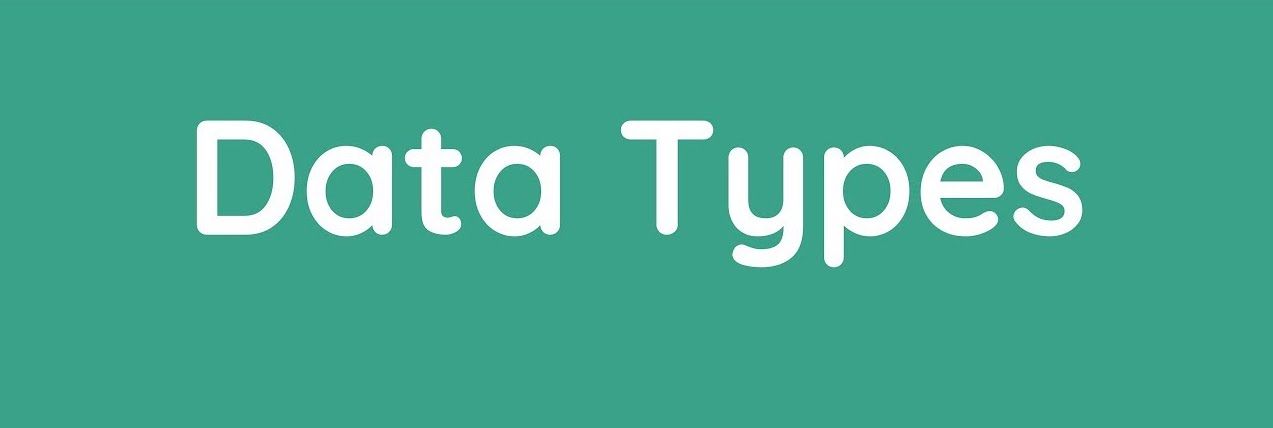
Representing Data
Data types (also known as data structures) allow programmers to represent information from the real world in code. They can be divided into 2 groups: primitive data types and complex data types. The specific types we'll focus on are:
Primitive Data Types
- Strings
- Numbers
- Booleans
Complex Data Types
- Arrays
- Objects
Primitive Data Types
Primitive Data Types essentially are data types that are "as simple as it gets". As you learn about string, numbers, and booleans below, try to think "is it possible to break this data type into smaller parts?" The answer is no, and hence they're considered the building-blocks of data in most programming languages.
The reason we make a distinction between "primitive" and "complex" data types has more to do with understanding mutability and different value types in JavaScript. For now you don't need to worry too much about this distinction, but if you're curious you can check out our post on mutability and value types.
Strings
A "string" is a set of alphanumeric characters strung together, often with the intent of saving data in a human-readable format (although not exclusively for that reason).
For example, if I want to represent a person's first name, I would use a String data type. (Think: I would have a hard time using numbers or true
or false
(booleans) to represent the name "Jim", right? So a string is the natural choice).
var firstName = "Jim";
Anything inside of a pair of single ' '
or double " "
quotation marks is a string in JavaScript. So even if I had var myNum = "42"
, the variable myNum
is still a string as far as JavaScript is concerned.
Numbers
A number is self-explanatory. A number is good for anytime you need to represent numerical data, especially if you anticipate ever needing to do math on that data.
var age = 42;
// When there's a birthday:
age++;
console.log(age); // 43
Sometimes choosing a data type won't be quite so obvious:
var zipCode = "90210"
// OR
var zipCode = 90210
Why not choose a number for zip codes? You definitely could, and that might make things easiest for you in your circumstances. But since you'll likely never have to do any math on a zip code, you should be just as safe saving it as a string. AND, perhaps one day you decide you want to include the full 9-digit zip code:
var zipCode = "90210-1234"
// if zipCode were a number, JavaScript thinks you want
// to subtract, so zipCode is now 88976...
var zipCode = 90210-1234; // oops...
Booleans
Booleans are simple - there are only 2 options: true
and false
. (Unlike numbers and strings where there are infinite possibilities)
These are perfect for representing dichotomous data - something that is either one possibility or the exact opposite of that possibility. E.g.: whether a user is an admin to your site or not, whether someone has a key to the building or not, whether something at a store is on sale or not.
var saleIsRunning = true;
var personIsAlive = false;
var isAdmin = true;
// etc.
Again, we could technically choose a string or a number to represent this kind of data, but there are a few problems with this:
- It isn't as intuitive
- It is more prone to error. Perhaps one programmer uses the string "yes" to represent whether someone is an admin, but another programmer uses "YES" or "Y", which are technically different strings altogether
- Conditionals (if statements) may react in unpredictable ways
Complex Data Types
Complex data types are simply a way to collect primitive data types together to help us handle more complex situations where data is involved.
Arrays
Arrays help us collect multiple other data types together. They're best used as a "collection of many things", and in JavaScript they can contain any combination of other primitive or complex data types. I.e.: an array can consist of strings, numbers, booleans, functions, objects, and even other arrays.
While some will tout JavaScript's flexibility to contain multiple different types of data inside a single array, this is almost always a bad idea. If you find yourself trying to create an array of more than just one data type, you're probably better off using an object instead of an array. See example below.
Arrays are created with square brackets: []
. If I set a variable to an empty set of square brackets, we call it an "empty array".
// An empty array:
var things = [];
// A useful array of strings. Collects together strings representing colors of the rainbow
var colors = ["Red", "Orange", "Yellow", "Green", "Blue", "Indigo", "Violet"];
// A collection of business expenses, which can now be easily totaled to a sum.
var expenditures = [109.99, 28.79, 29.95, 224.95];
// A bad example for the use of an array. Should use an object instead:
var person = ["Sam", "Johnson", 30, "brown", "blue"];
// ("brown" hair and "blue" eyes, but that isn't obvious from using an array)
Array Index
Items in an array can be accessed later by referring to their index number. Items in an array are automatically assigned a number (starting with 0) of where that item is stored in the array.
var myArray = ["a", "b", "c", "d", "e", "f"]
// index: 0 1 2 3 4 5
To access an item in an array, use square brackets after the name of the array, with the index of the item you're trying to access:
var myArray = ["a", "b", "c", "d", "e", "f"];
console.log(myArray[0]) . // logs "a"
console.log(myArray[3]) . // logs "d"
console.log(myArray[5]) . // logs "f"
Nested Arrays (Matrix)
We mentioned you can have an array inside of an array. Accessing items of the inner array is logical - use 2 pairs of square brackets after the array's variable name:
var myArray = [
["a", "b", "c"],
[true, true, false],
[42, 43, 44]
]
console.log(myArray[0][2]) // logs "c"
console.log(myArray[2][2]) // logs 44
If you'd like to read a little more, check out our writeup on Arrays
Objects
Where arrays are a way to represent a collection of many things, objects are great for representing one thing in a lot more detail.
For example, if I want to save information about a person in my program, I could save them as a string:
var person = "Sarah";
But I only have a small piece of information about that person - their first name. Maybe I want to save their last name, age, hair color, eye color, and whether they're a cool person or not. This is the perfect time to use an object:
var person = {
firstName: "Sarah",
lastName: "Smith",
age: 26,
hairColor: "Red",
eyeColor: "Green"
}
In the above example, firstName
, lastName
, age
, hairColor
, and eyeColor
are called properties (or sometimes "keys") of the person
object. "Sarah"
, "Smith"
, 26
, "Red"
, and "Green"
are called the values of those properties.
Accessing properties of an object
To grab the value of a property of an object, there are two methods you could use. The most common is to use dot notation, where you type the name of the object (person
in the above example), followed by a dot (.
), and then the property name you're trying to access:
console.log(person.firstName); // logs "Sarah"
console.log(person.age); // logs 26
console.log(person.favoriteColor); // logs undefined - our object doesn't have that property
Another, less common (but sometimes necessary) way to access values from an object is to use a syntax similar to how we accessed items from an array, using square bracket notation. Only with an object, since objects don't have an index for each item, we use the string version of the property name:
console.log(person["firstName"]); // logs "Sarah"
console.log(person["age"]); // logs 26
console.log(person["favoriteColor"]); // logs undefined - our object doesn't have that property
For now, just get used to using dot notation. Later we'll run into instances where you'll be required to use the bracket notation instead.
Conclusion
There is a lot more to learn about each of these data types. For now, this overview should give you a good start to be able to understand how you might take data from the real world and represent that data in a computer program.