RPG
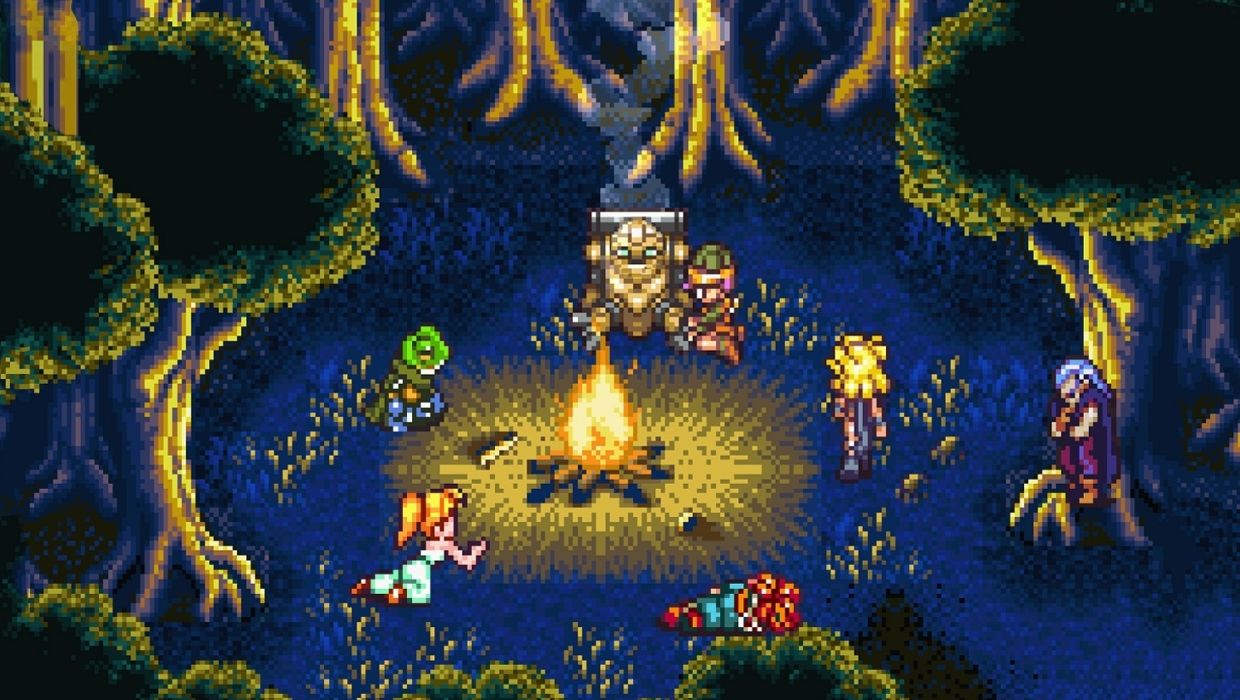
This homework will take a while so get started as soon as possible.
You are going to create a RPG web page.
This is how it will work:
- When the page loads or refreshes:
- The player will enter their name
- The player will choose between 3 characters (images)
- After they enter a name and select a character a
Start Game
button will become active and can be clicked to start game
- When the Start Game Button is clicked:
- A random enemy will appear on the right-hand side (there are 3 enemies that can be picked from
- It will show the enemy's name and current health
- An attack button will appear under your character
- When you click the attack button text will show somewhere on the screen saying, "You attacked [enemy name] for [x] damage" where enemy name is the name of the current enemy and where x is how much damage you hit the enemy for.
- Player damage should be randomly calculated each time
- Player should have a 1 in 3 chance of missing the attack. If missed the text will say, "[player name] attempted attack against [enemy name] but missed"
- After you attack your attack button is disabled while the enemy makes his attack - similar text will appear saying "[enemy name] attacked [player name] for x damage". Each enemy can have random damage or a preset damage amount.
- Enemy should have a 1 in 3 chance of missing the attack. If missed the text will say, "[enemy name] attempted attack against [player name] but missed"
- If the player kills an enemy the text on the screen should say "[player name] killed [enemy name]".
- After enemy is killed player should have a 1 in 2 chance of receiving a health potion. The health potion can have a pre-set or randomized heal power.
- If an enemy is killed the next enemy appears (there are 3 enemies total)
- After all 3 enemies are dead the player will win the game (have text on the screen that indicates so)
- After winning, a prompt will say, "Do you want to play again?" with a "Yes" button. If selected the game restarts (as if the page was refreshed).
Other requirements:
- Must be responsive (Use Bootstrap. If you are expert at CSS then you don't have to use Bootstrap. Otherwise use Bootstrap)
Extra Credit
- Add sound effects for everything
- Add different player weapons that can be used and obtained
- Allow player to control multiple characters who each get a turn to attack (Similar to Final Fantasy
- Allow multiple enemies at the same time to be fought each who get a turn to attack (Similar to Final Fantasy)
Graphics you can use (non-commercial only):
End result could look like this:
Or like this:
Hints:
- Functions Refresher
- Objects Refresher
- Use constructor functions as objects:
var Enemey = function() {
var enemyNames = ['Weak Skeleton', 'Fierce Demon', 'Ugly Wargile'];
var generateHp = function () {
//write code to generate random hp
//return random hp
};
var generateName = function() {
//grab a random name from the enemyNames array and set it
};
this.hp = generateHp();
this.name = generateName();
}