PropTypes
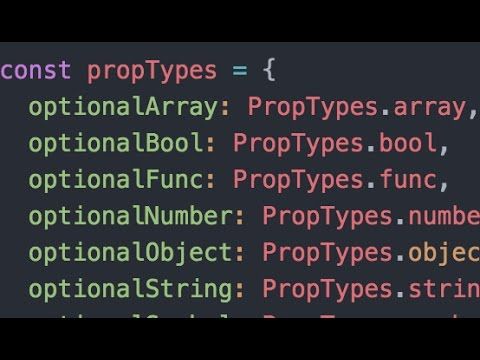
https://reactjs.org/docs/typechecking-with-proptypes.html
Type Checking
Type checking is an important principle in programming.
JavaScript lets you get away with a lot of potentially hazardous coding practices. Consider the following:
When you code let a = 1
and then let a = 2
, JavaScript yells at you and breaks. But when you code var b = 1
and then var b = 2
, JavaScript lets you get away with it.
var
use to be our only option. Why would we want to use let
when it's more likely to break our code?
It's because it breaks it early instead of later, becoming a deeper issue. We're able to say, "Oh yeah! I did want that variable to be this. Thank you for breaking my code and telling me later instead of me being confused that my variable values aren't what I expect them to be"
With PropTypes, we are able to say, "I expect this prop to be a string, and this one to be a number, and if it's not, let me know."
Installation
PropTypes are not part of React, but are extremely common. If you used Create React App, you already have it and you just need to
import PropTypes from "prop-types"
at the top of any file you're using it in.
If you don't have it, you'll need to install it too.
npm install --save prop-types
Now at the bottom of a component file that is expecting to receive props, you will name the component and assign PropTypes by giving that component another property called propTypes
.
import PropTypes from 'prop-types'
class Greeting extends React.Component {
render() {
return (
<h1>Hello, {this.props.name}</h1>
<h2>You are {this.props.age} years old</h2>
)
}
}
Greeting.propTypes = {
name: PropTypes.string,
age: PropTypes.string
}
Here, we are saying, "I am expecting the name
prop to be a string."
Check the docs for what else you're able to expect a prop to be:
https://reactjs.org/docs/typechecking-with-proptypes.html
Options include:
- array
- boolean (bool)
- function (func)
- number
- object
- string
- symbol
- node (Anything that can be rendered: numbers, strings, elements or an array)
- element (A React element)
You're also able to make sure an object fits a specific shape:
ComponentName.propTypes = {
NameOfPropThatIAnOptionalObjectWithSpecificShape: PropTypes.shape({
color: PropTypes.string,
fontSize: PropTypes.number
})
}
And make sure things are required:
requiredString: PropTypes.string.isRequired,
You are also able set default prop values. Which is hecka cool.
Greeting.defaultProps = {
name: 'Stranger'
}
So, if you don't want to pass in a prop, that component will receive a prop. And that that prop can easily be overwritten if you want it to be.
Start using PropTypes in your components. You'll write much more manageable code if you do, and many companies require it.