Practice Problems
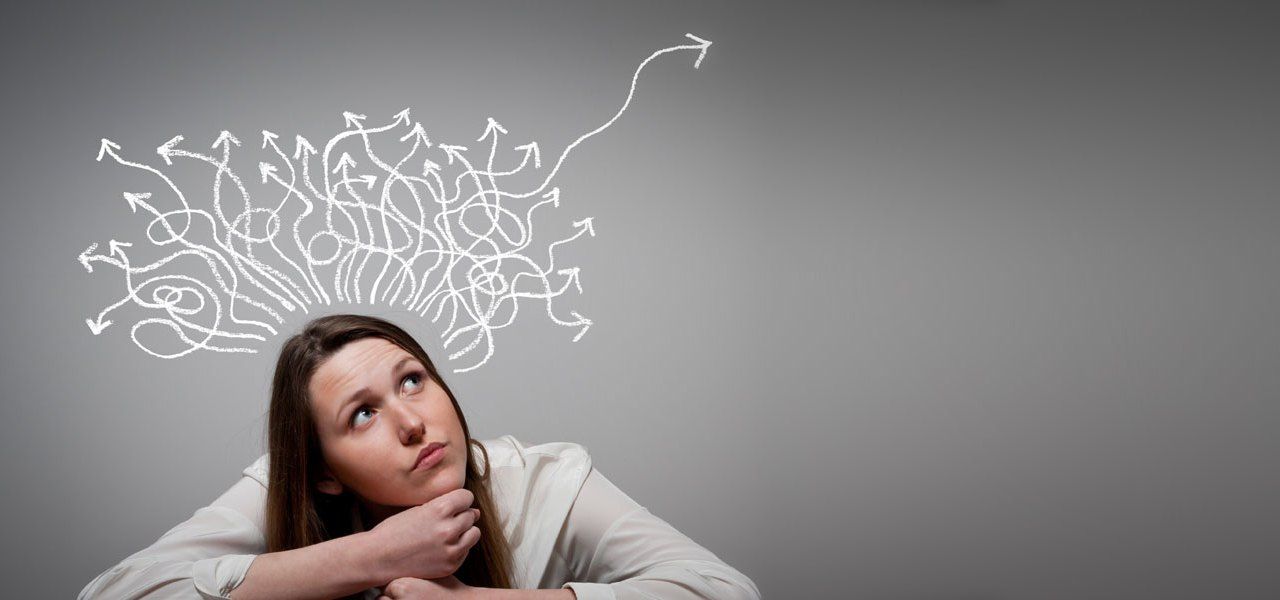
Introduction
Now that you have a basic understanding of the building blocks that make up JavaScript you are going to use them in problem-solving.
- Make a function that takes a string and returns that string reversed.
Example
Input: "Hello World"
Output: "dlroW olleH"
let reverseStr = function(str) {
}
- Make a function that takes a string and returns true if the string could be a number else return false.
Example
Input: "3"
Output: true
Input: "three"
Output: false
let isNum = function(str) {
}
- Make a function that takes a number and returns true if the number is even else return false.
Example
Input: 4
Output: true
Input: 3
Output: false
let isEven = function(num) {
}
- Make a function that takes an array and returns the average of the array.
Example
Input: [1, 2, 3]
Output: 2
Input: [5, -10, 10, 20]
Output: 6.25
let averageArray = function(arr) {
}
- Make a function that takes two arrays and returns a single array of the items from the arrays added alternatingly.
Example
Input: ["a", "b", "c"] and [1, 2, 3]
Output: ["a", 1, "b", 2, "c", 3]
let combineArrays = function(arr1, arr2) {
}