JSX
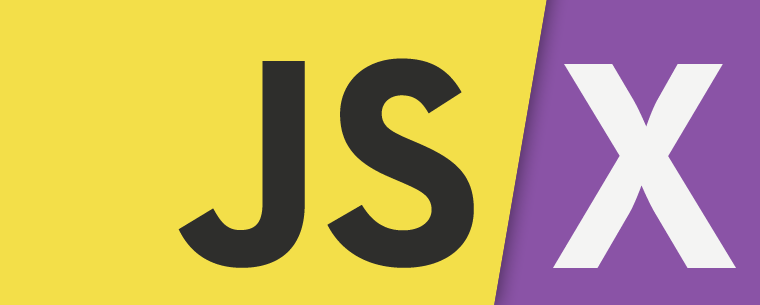
JSX
JavaScript and XML
JSX is Javascript and XML. By this point in the class you should know JavaScript but what is XML? To understand it let's look at HTML VS XML.
HTML
Hyper Text Mark Up language
<h1>
<p>Hello World</p>
</h1>
XML
eXtensible Markup Language.
<anything>
<clownShoes> Hello World </clownShoes>
</anything>
The difference here is the 'extensible' part of XML. HTML has defined tags while in XML you can have any tags.
So when understanding JSX you need to know JavaScript and that you can now have any tags that you like those include the old HTML tags such as h1, p and new custom tags that you will make in React.
We will use JSX within React. We will write code that will say, "Use these HTML tags with sometimes slightly altered attribute names, and we can also pass easily pass JavaScript in as attributes."
<div className="red">Children Text</div>;
<MyCounter count={3 + 5} />;
// Here, we set the "scores" attribute below to a JavaScript object.
var gameScores = {
player1: 2,
player2: 5
};
<DashboardUnit data-index="2">
<h1>Scores</h1>
<Scoreboard className="results" scores={gameScores} />
</DashboardUnit>;
Notice className
is a replacement for class
. This is because class is an ES6 reserved word.
In this example, we also have a <Scoreboard />
tag. This isn't a regular HTML tag, but is a reference to a class/constructor that we will build somewhere else. We can pass in attributes to that class with the scores={gameScores}
syntax.
The above code gets compiled down to:
The above gets compiled to the following without JSX. I hope you will agree JSX syntax reads more naturally.
React.createElement("div", { className: "red" }, "Children Text");
React.createElement(MyCounter, { count: 3 + 5 });
React.createElement(
DashboardUnit,
{ "data-index": "2" },
React.createElement("h1", null, "Scores"),
React.createElement(Scoreboard, { className: "results", scores: gameScores })
);
If you are sane, you prefer the first code snippet.
A Couple of Rules
-
Since JSX compiles into calls to React.createElement, the React library must also always be in scope from your JSX code.
-
User-Defined Components Must Be Capitalized. This is why
<Scoreboard />
is capitalized. -
You can only return one JSX element. This means if you want to return two, you need to wrap them in a parent. Usually a
<div />
-
When using inline event listeners like onclick right them in camelCase (onClick)
<div>
<MyFirstComponent />
<MySecondComponent />
</div>
For some more depth, the React Docs do an exellent job of talking about JSX simply but in depth. React JSX DOS