Intro to React
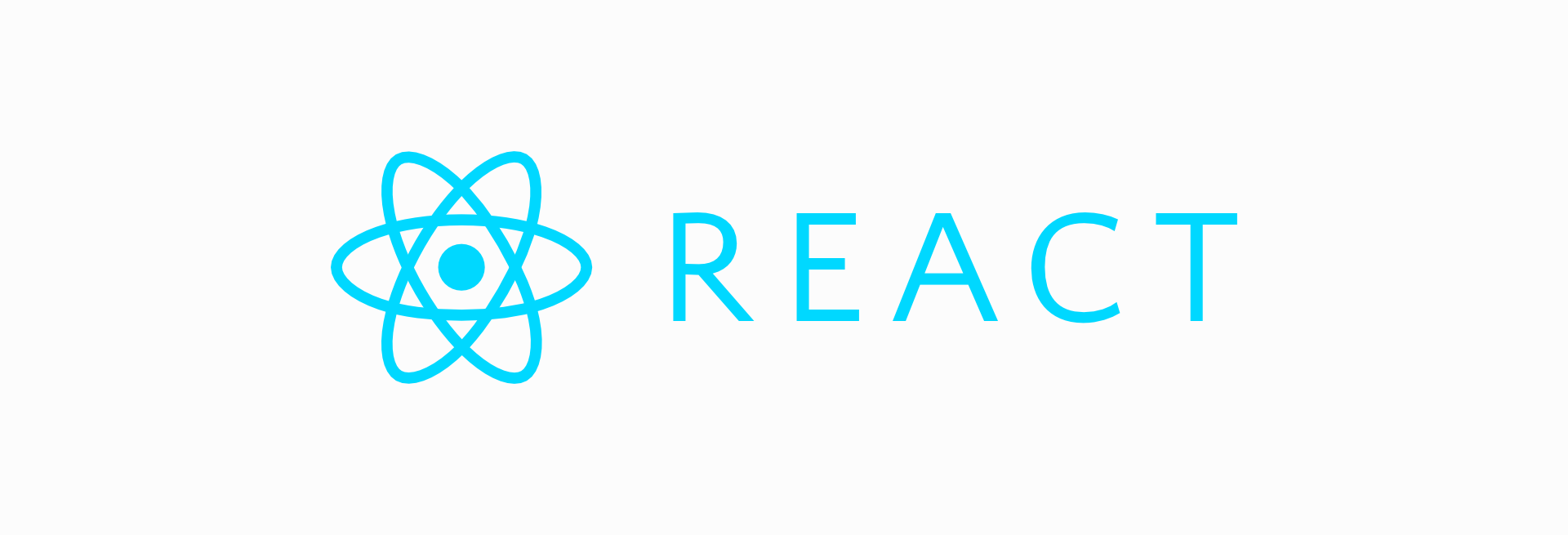
What is React?
React is a lightweight, fast, front-end library created by Facebook that enables developers to create component-based front end applications. It emphasizes one-way data binding and uses a new tool called the "virtual DOM" to minimize rendering.
Why React?
React enables developers to easily create reusable, fully-self-contained components. This allows developers to extend the HTML vocabulary by creating their own reusable HTML elements. This seemingly simple addition means that developers can build their software out of higher-level components that are defined once and can be reused across the application's code.
React makes it simple to express how your app should look at any given point in time, and React will automatically manage all UI updates. React manages state (your application's data) safely by reducing the potential for unwanted side effects and glitches when data is changed.
React is very fast, primarily due to its use of the "Virtual DOM". Before React, making changes to the DOM was a very expensive (slow) thing to do. React's use of the Virtual DOM makes it an extremely fast way to render elements on a page, especially when changes to the DOM are required.
How does React work?
To better understand how the Virtual DOM works, check out this short video explanation:
React maintains two versions of the DOM: the Virtual DOM and the actual DOM that is displayed to the user. When data changes, instead of rendering the page, or an entire section of the page, React updates the virtual DOM and then compares it to the actual DOM. Then, only the things that are different get changed. DOM manipulation is one of the slowest parts of web development, and React minimizes this.
Declarative
React is "declarative", which means you as a developer get to write your programs in a way that tells the computer what to do, rather than how to do it. In plain DOM JavaScript, if you wanted to pull the text from an input box and put it on the screen, you'd end up writing something like this:
var todoList = document.getElementById("todo-list");
var newTodo = document.createElement("li");
var newTodoText = document.getElementById("new-todo").value;
newTodo.appendChild(newTodoText);
todoList.appendChild(newTodo);
Explicitly telling the computer, step-by-step how to do something is typically a long-winded way to write code. With React, you get to trust that React knows how to tell the computer to do all these steps, and you get to instead tell React what to do by using regular old, pure JavaScript.
Component-based architecture
Think back to some past web sites you've worked on in vanilla HTML. You likely divided the HTML files where each file represented a page of the website. And if it was a substantial website, each of those files was potentially hundreds and hundreds of lines long. Even if you did a good job of using semantic HTML5 tags, it was probably a nightmare to try and find any particular part of the web page.
Component-based architecture allows the developer to essentially create his/her own HTML tags! This way, instead of having an index.html
file that uses the bootstrap navbar look like this (you don't need to read through this):
...
<body>
<nav class="navbar navbar-default">
<div class="container-fluid">
<!-- Brand and toggle get grouped for better mobile display -->
<div class="navbar-header">
<button type="button" class="navbar-toggle collapsed" data-toggle="collapse" data-target="#bs-example-navbar-collapse-1" aria-expanded="false">
<span class="sr-only">Toggle navigation</span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
<a class="navbar-brand" href="#">Brand</a>
</div>
<!-- Collect the nav links, forms, and other content for toggling -->
<div class="collapse navbar-collapse" id="bs-example-navbar-collapse-1">
<ul class="nav navbar-nav">
<li class="active"><a href="#">Link <span class="sr-only">(current)</span></a></li>
<li><a href="#">Link</a></li>
<li class="dropdown">
<a href="#" class="dropdown-toggle" data-toggle="dropdown" role="button" aria-haspopup="true" aria-expanded="false">Dropdown <span class="caret"></span></a>
<ul class="dropdown-menu">
<li><a href="#">Action</a></li>
<li><a href="#">Another action</a></li>
<li><a href="#">Something else here</a></li>
<li role="separator" class="divider"></li>
<li><a href="#">Separated link</a></li>
<li role="separator" class="divider"></li>
<li><a href="#">One more separated link</a></li>
</ul>
</li>
</ul>
<form class="navbar-form navbar-left">
<div class="form-group">
<input type="text" class="form-control" placeholder="Search">
</div>
<button type="submit" class="btn btn-default">Submit</button>
</form>
<ul class="nav navbar-nav navbar-right">
<li><a href="#">Link</a></li>
<li class="dropdown">
<a href="#" class="dropdown-toggle" data-toggle="dropdown" role="button" aria-haspopup="true" aria-expanded="false">Dropdown <span class="caret"></span></a>
<ul class="dropdown-menu">
<li><a href="#">Action</a></li>
<li><a href="#">Another action</a></li>
<li><a href="#">Something else here</a></li>
<li role="separator" class="divider"></li>
<li><a href="#">Separated link</a></li>
</ul>
</li>
</ul>
</div><!-- /.navbar-collapse -->
</div><!-- /.container-fluid -->
</nav>
<script src="index.js"></script>
</body>
...
You can create a separate <Navbar>
component that allows your index.html
to looks like this instead:
...
<body>
<Navbar />
<script src="index.js"></script>
</body>
...
The <Navbar>
component still needs to be defined somewhere, so you would make a separate file dedicated to just the navbar. The nice thing about organizing it this way is that you can now reuse the Navbar component you just made across multiple pages without having to copy/paste the long code. AND if you need to make a change to the navbar, you can do it in just one place instead of every place the navbar was copy/pasted!
Unidirectional Data Flow
Some earlier front-end web frameworks like Angular 1 would automatically provide something called "two-way data binding." All you need to know about it is that it could automatically update what you see on the page based on every single change in data. Remember how updating the UI is the slowest part of what gets done on the web? This tended to make Angular a very heavy, slow framework.
While you can mimic this behavior in React, the default is to emphasize unidirectional data flow. Essentially this means that when the state (data) updates, it will wait until you explicitly tell it to update the UI. This way, React can be as efficient as possible when it comes to updating the view.
Conclusion
A lot of the above will make more sense as you learn more about React. React is currently one of the hottest frameworks out there for developing the front end, and is even make headway into creating native mobile applications with React Native. Its maintenance by Facebook and its support from the community make it one of the best, most performant frameworks available today.