Intro to JavaScript
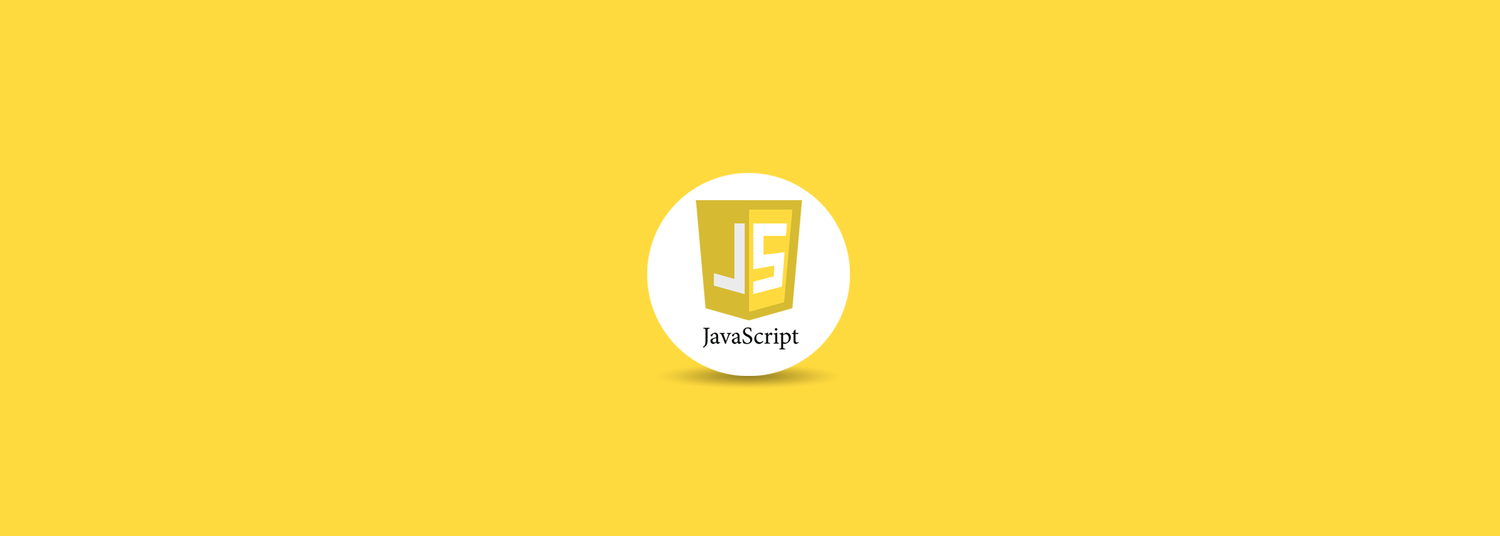
History of Javascript
JavaScript was originally created in 10 days by Brendan Eich who was working at Netscape. The language is often confused by non-developers with Java, but they actually have very little in common. Those pushing JavaScript received a trademark license from Sun, but before that the language was ECMAScript. ECMAScript didn't stick partially because of a lack of cooperation from Microsoft and support in their product Internet Explorer.
Who Uses JavaScript?
Pretty much everyone! If you're on a website that was made sometime after about the year 2000, that website probably uses JavaScript. Any website that has any level of user interaction, google analytics tracking, cookies or local storage, etc. is using JavaScript to make the user experience better and more interactive.
Where is JavaScript used?
Originally, it was only ever used as a way to control a website's behavior. Every browser comes with a little JavaScript engine, whose job it is to read and execute JavaScript code that is tied to an HTML page through the HTML <script>
element. So when the browser is reading this code:
...
<body>
<h1>Hello world!</h1>
<script src="app.js"></script>
</body>
...
it reads it from top-to-bottom. As soon as it sees the <script>
tag, it stops reading HTML and tells the JavaScript engine to read through all the JavaScript code found in the app.js
file.
That code may be in charge of modifying how things get displayed on the page (beyond whatever CSS is doing), handling certain events that the user (or the browser) may initiate (such as what should happen if a button is clicked), and so forth.
For many years, this was the only way to run JavaScript - you'd have to build an HTML page, link your JavaScript file in the HTML via the <script>
tag, then open that HTML page in a browser and use the dev tools to see what JavaScript was doing. (To see these tools, right click on any web page and choose "inspect", then click on the "console" tab).
Fast forward to 2011. Google had built the world's best JavaScript engine into its Chrome browser and open-sourced the code. That open-sourced engine was then used to create node.js, which forever changed the future of JavaScript as a language.
It may seem small, but node.js made it so JavaScript no longer needed to be written in the context of a web page. Instead, it turned it into a language that could also be run from the terminal. This means that JavaScript coders could now write server-side scripts, allowing them to write both client-side (browser) and server-side (server) applications all in JavaScript!
Main takeaway: there are two ways you'll be running your JavaScript code: 1) in the context of an HTML document, where you use the
<script>
tag to link it to your HTML file, and 2) inside the terminal using node.js to run the code. There are some important nuances to understand between these two different ways, and you'll become familiar with them in the coming weeks.
Basic JavaScript Terminology
Statements
Statements are the instructions of a computer program – they are executed by the computer and perform some action or computation. Each statement in JavaScript is ended with a semicolon. Statements are composed of values, operators, expressions, keywords, and comments.
var dog = "Sparky";
console.log(dog + "! Come back here!");
myFunction();
Each of the above lines of code is a separate statement.
Values
There are two types of values that JavaScript defines: Fixed values and variable values.
Fixed values are called literals.
Variable values are called variables.
Literals
We'll spend lots of time on each of these later, but here's a quick rundown of the most important data types in JavaScript:
Numbers are written with or without decimals (but no commas), like:
700.505
384
Strings are text, written within double or single quotes:
'V School is the best.'
"I'm learning to code!"
'I\'m learning to code!'
Note: Strings get their name from the fact that it is a string of characters, i.e. a bunch of characters strung together.
A boolean is either the value true
or the value false
, without quotes around it (otherwise it would just be a string).
An array is a collection of other types of data. Array literals are defined with a set of square brackets surrounding the items that are part of the array:
var myThings = ["headphones", "laptop", "phone"];
var expenses = [10.99, 2.95, 34.95]
An object is another form of collection, like arrays. Only objects are used to describe a single thing in more detail rather than collect many things like an array:
var car = {
make: "Tesla",
model: "X",
year: 2016
}
Variables
Variables in any programming language are used to store data values for a later time. JavaScript uses the reserved keyword var
to define variables.
An equal sign (=
) is called the assignment operator and is used to assign values to variables.
To use a variable you must first define it, then you must assign it, then you can use it anywhere in your code.
Always remember you must:
- Define it.
- Assign it.
- Use it.
Always in that order.
If you want, you can do steps 1 and 2 separately:
var myVariable;
myVariable = "My literal string.";
Or together in the same line:
var myVariable = "My string literal."
A variable can be assigned to equal anything. To compare to the physical world we live in, imagine it as a box that holds 1 thing. You can also imagine it as the label on a file in a filing cabinet - you can get the contents of the file only if you know what name it's filed under.
Here are some examples of declaring variables to several different things...
// a string
var myName = 'Peter Parker';
// a number
var myAge = 28;
// an array
var myJobs = ['Daily Bugle Reporter', 'Spider-Man'];
// an object
var myEducation = { 'university': 'Empire State University', 'major': 'Science' }
// a function
function getBio() {
return myName + " is " + myAge + " years old.";
}
Operators
JavaScript uses the assignment operator - single-equals sign (=
) - to assign values to variables, as we just saw above.
var myVariable = 78;
var yourVariable = 10;
Other common operators include the arithmetic operators:
var x = 9;
var y = x * 5;
var z = x + 2*y;
Reserved Words In JavaScript
Reserved words are words that the compiler will look for when interpreting your code and has attached special meaning to. You can't use these words as the name of your variables or functions or it will cause an error.
Comments
// This is a one-line comment
/* This is a multiple line
(or block)
comment */
Functions
A function is not the same thing as a variable. A function is a named procedure that performs a specified service.
The best kinds of function do one thing and they do it well.
Just like a variable, a function must be declared and defined in order to be used. This is usually done in the same line. You will use the reserved keyword function
when you declare a function.
function myMultiplyFunction(arg1, arg2) {
return arg1 * arg2;
}
The above function uses the return
reserved keyword to specify what value the function should give back to the rest of the code after it has finished.
Because you can declare a variable and set it equal to an anonymous function -- or a function without a name -- then the above function can also be written as...
var myMultiplyFunction = function(arg1, arg2) {
return arg1 * arg2;
}
In order to use a function you must call it. You call it by its name, followed by a set of parentheses, including any parameters that function requires.
You can call the above functions by writing the following line of code...
myMultiplyFunction(5,7);
Packages and NPM
There are many problems that are presented to developers that are universal. These problems have already been solved by brilliant programmers, and we'd like to take advantage of those solutions.
Most languages have many pieces of third party software that are solutions to those problems. A package could help you with a very simple task or could be the framework that you decide to build your project in. Your projects will probably utilize many of these helpful packages.
This software can be made by individuals, organizations, or corporations.
It's extremely nice to have a package manager to help organize all of these packages within your project. The most popular JavaScript package manager is NPM(Node Package Manager).
Once you have NPM installed (it comes with node), you can install a package to your project folder. But before you want to initialize your own project as a node package. Do so with:
npm init -y
If you leave off the -y
you will either have to manually except the default setup (which are some good defaults), or put in your own. The -y
stands for "yes to all".
This will also build your package.json
file. This file will tell NPM what do with with different commands like npm install
or npm start
.
Now you can install a package with:
npm install new-package --save
Where "new-package" is the name of the package.
--save
adds that package and version to your package.json
.
to install a package globally
, or in other words, accessible wherever on your computer, just add a -g
.
npm install -g new-package
For packages that you are using to help you write code, you'll want to install them locally, for packages that you are using to help you run code, you'll want to install them globally.
When you install a package locally, it will store them in a folder called node_modules. This is an important folder to add to your .gitignore
.
Some popular packages right now are:
- lodash
- react
- jquery
- body-parser
- webpack
- express
- redux
- mocha
- chai
- mongoose
This is barely scratching the surface. The more packages you are familiar with, the more valuable of a JavaScript developer you will be.
Conclusion
There's a ton you're going to be learning about all of this, but this should give you a good set of terminology to study up on and become familiar with.