Intro to Flux
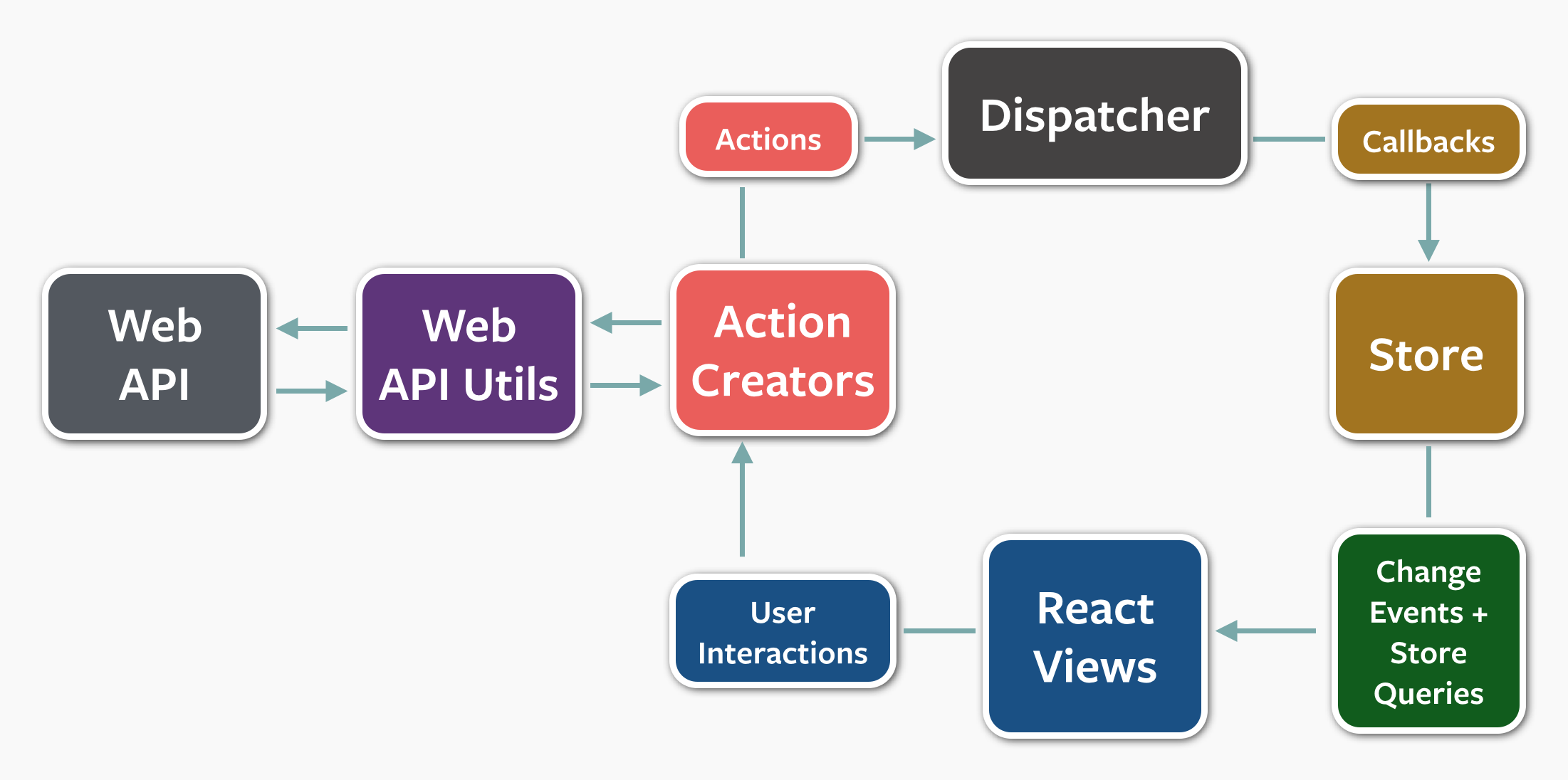
Introduction
To understand Flux we first need to understand where Flux came from. Flux evolved from "MVC" (short for Model, View, Controller), which is a design pattern used to make web-based interfaces. In short, organizing your code is hard, and MVC is one very popular philosophy on how to do it right.
What is MVC?
MVC (Model, View, Controller) is a way of organizing pieces of your code in a logical way. Below you'll find descriptions of each part of MVC:
Model
Data (either from the database or data stored locally). Only the Controller can retrieve data and make modifications to the Model.
View
Displays the data to the user and allows the user to interact with the application. The View can't interact directly with the Model.
Controller
Controls the interaction between the Model and the View. In other words, the Controller is in charge of deciding how the Model should be displayed and how to process an action from the View (user) to modify the Model.
Why use it?
The MVC design pattern allows for better development and easier debugging since responsibilities are clearly separated, but it also adds more overhead when building projects.
React with MVC
React is technically considered to be the "View" in the MVC structure but due to complications with MVC, Facebook also released their own architecture called Flux. Instead of having two-way data binding from the controller to the view, Flux uses one-way data flow. Two-way data binding was difficult to debug as it could have many side effects on your application. Using one-way data flow allowed for easier debugging and scaling with larger applications.
What is Flux?
Flux is made up of four different parts.
1) Actions
An object with data about the action that just occurred on the application.
2) Store
Contains the application state (data) and logic.
3) Dispatcher
Processes action and their callbacks
4) View
Renders out to data to the screen and listens to changes in the store to re-render.
Notice how the arrow is never going two directions - it's always one-way.
Summary
Flux is similar to MVC in some ways but uses one-way data flow to simplify application development. It works by having a store that passes data to the view which renders it to the screen. An action in the application goes to the dispatcher which passes it the store that processes the data and then, in turn, passes the updated data to the view.
Next step learn about Redux the most popular implementation of flux.