Inline Styling with React
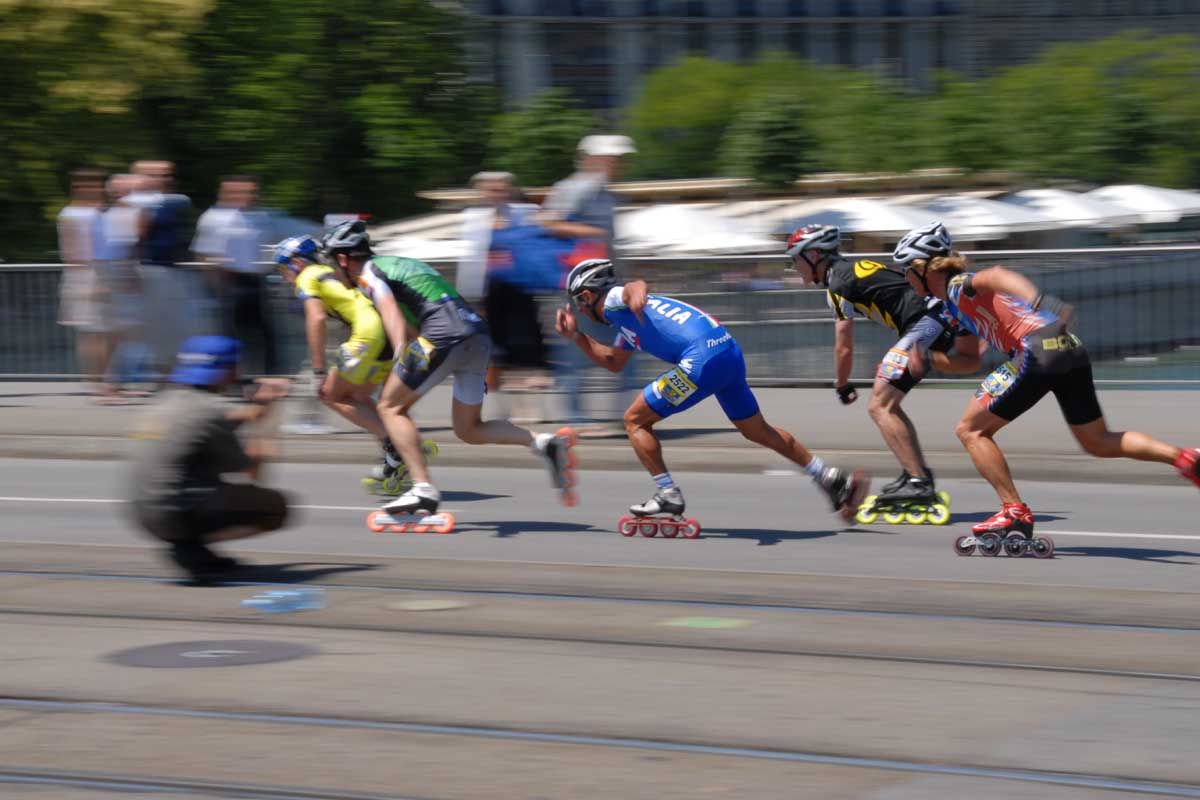
Intro
If you want to pass style down through props, are building animations, or want some conditional styling in React you will find yourself in need of inline styling
.
In React, inline styles are not specified as a string. Instead, they are specified with an object whose key is the camelCased version of the style name, and whose value is the style's value, usually a string.
Example:
import React from "react";
class Hello extends React.Component {
constructor() {
super();
this.style = {
color: "red",
fontSize: "10px",
textAlign: "center"
};
}
render() {
return (
<div style={this.style}>
Hello, it's me. I was wondering if after all these years you'd like to meet.
</div>
)
}
}
export default Hello;
Note on combining multiple inline style objects
If you have two objects with style that you want an element to use. You would use the spread operator like so.
<button style={{...this.styles.button,...this.styles.backButton}}>Back</button
Passing Style Down With Props
Sometimes you define your style in a parent component. If you do this you must use props to access the styling in the child.
Example:
// ./components/box/Box.js
import React from "react";
class Box extends React.Component {
render() {
return (
<div className="box" style={this.props.style}>
<div>
)
}
}
export default Box;
// ./App.js
import React from "react";
import Box from "./components/box/Box.js
class App extends React.Component {
constructor() {
super();
this.style = {
color: "red",
borderColor: "orange"
};
}
render() {
return (
<div>
<Box style={this.style} />
{/* OR */}
<Box style={{color: "purple", borderColor: "orange"}} />
</div>
)
}
}
export default App;
Usage with State
Now that we know how to use inline styles we can change those based off of the current state. We can either directly set styling values in state or you ternary operators to toggle values.
CSS as State
When saving CSS as state we set are initials styles in the constructor and make custom methods that change the state. We then set the element's style equal to this.state or this.state.< where CSS is saved >
import React from "react";
class App extends React.Component {
constructor() {
super();
this.state = {
backgroundColor: "blue",
height: "100px",
width: "200px"
}
this.changeColor = this.changeColor.bind(this);
this.changeWidth = this.changeWidth.bind(this);
}
changeColor(backgroundColor) {
this.setState({
backgroundColor
});
}
changeWidth(width) {
this.setState({
width
});
}
render() {
return (
<div style={this.state}
onMouseEnter={() => this.changeColor("red")}
onMouseOut={() => this.changeColor("blue")}
onClick={() => this.changeWidth("400px")}
onDoubleClick={() => this.changeWidth("200px")}
/>
)
}
}
export default App;
Style with Ternary Operators
When using ternary operators for state we make a boolean comparison in the inline style and set what the value will be for true and for false. In are example below we are toggling a boolean value that will hide or show text.
import React from "react";
class App extends React.Component {
constructor() {
super();
this.state = {
isShowing: false,
}
this.toggleIsShowing = this.toggleIsShowing.bind(this);
}
toggleIsShowing() {
this.setState(prevState => ({
isShowing: ! prevState.isShowing
}));
}
render() {
return (
<div>
<p style={{display: this.state.isShowing ? "inherit" : "none"}}>This is the text!</p>
<button onClick={this.toggleIsShowing}>Show Text</button>
</div>
)
}
}
export default App;
Now you know how to use inline styling to make a dynamicily styled app!