HTML / HTML 5
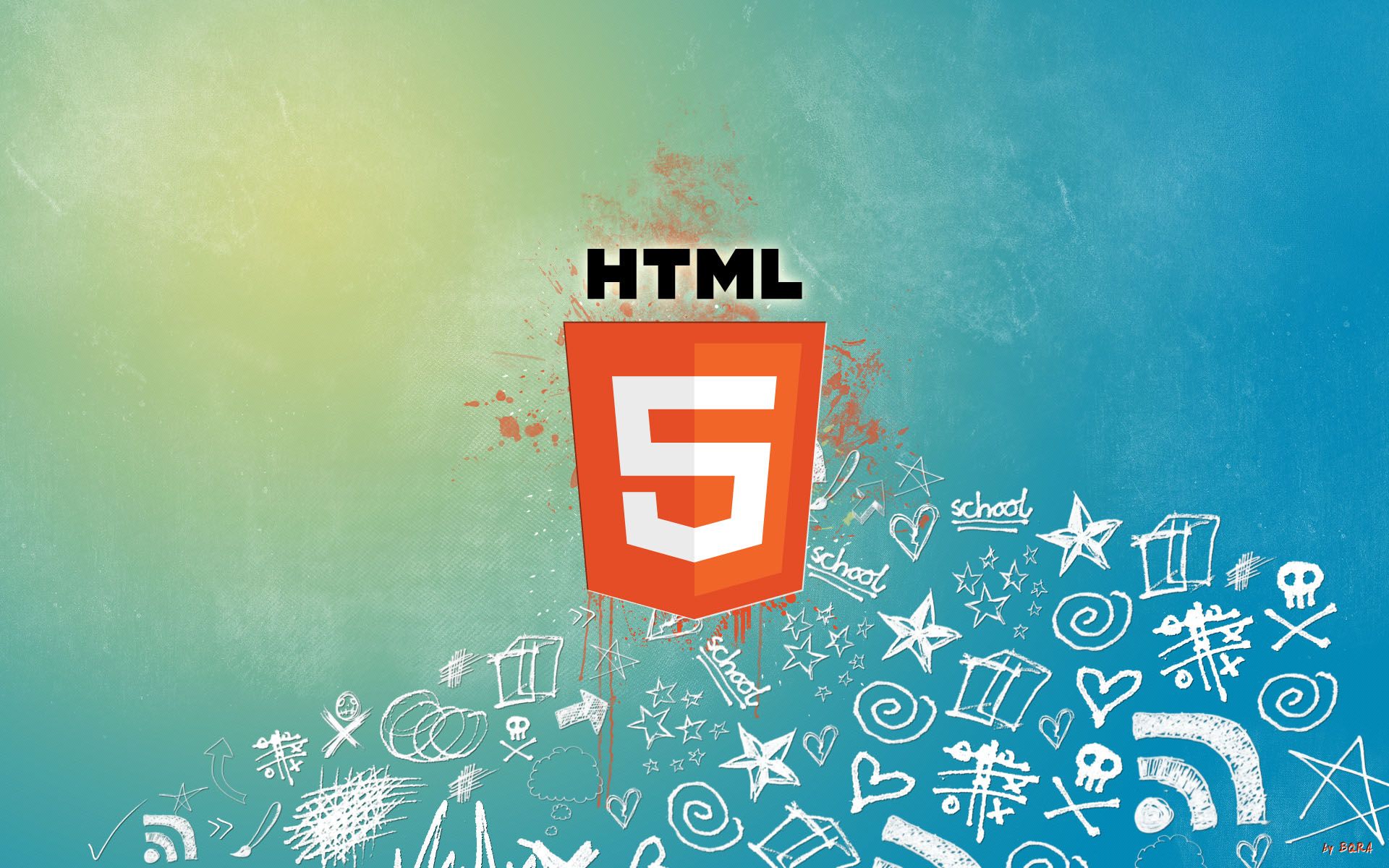
HTML is the skin and bones of the internet. HTML is used on every single website on the internet. By learning to use HTML you are setting a foundation of knowledge that is absolutely essential if you ever want to qualify for even the most junior of all web developer positions.
Vocabulary
HTML - Stands for Hypertext Markup Language, a standardized system for tagging text files to achieve font, color, graphic, and hyperlink effects on World Wide Web pages.
tag - An HTML tag is a code that describes how a Web page is formatted. HTML tags are defined by the characters <
and >
.
element - An HTML element is an individual component of an HTML document or web page, once this has been parsed into the Document Object Model. HTML is composed of a tree of HTML elements and other nodes, such as text nodes. Each element can have HTML attributes specified.
attribute - An HTML attribute is a modifier of an HTML element. They are usually added to a HTML start tag, which along with content and end tag comprises most HTML elements. Particular attributes are only supported by particular element types; if they are added to elements that do not support them they will not function.
block - Most HTML elements are defined as block level elements or inline elements. Block level elements normally start (and end) with a new line, when displayed in a browser. Examples: <h1>
, <p>
, <ul>
, <table>
, etc.
inline - Inline elements are normally displayed without line breaks. Examples: <b>
, <td>
, <a>
, <img>
, etc.
url - A URL is one type of Uniform Resource Identifier (URI); the generic term for all types of names and addresses that refer to objects on the World Wide Web. The term "Web address" is a synonym for a URL that uses the HTTP / HTTPS protocol.
HTML elements are written with a start tag, with an end tag, with the content in between:
<tagname>content</tagname>
HTML elements with no content are called empty elements.
<br>
is an empty element without a closing tag (the <br>
tag defines a line break).
Empty elements can be "closed" in the opening tag like this: <br />
.
HTML5 does not require empty elements to be closed. But if you want stricter validation, or you need to make your document readable by XML parsers, you should close all HTML elements.
Super Important Elements
Every web page has to have certain elements in order to work correctly.
Open up your favorite text editor application and create a new file. Save that file and name it whatever you'd like so long as it has .html at the end of it. The standard name for the main html file of a website is "index.html".
Now you should have an empty html file to work in.
The elements every page has to have are html
, head
, body
.
The HTML element is everything from the start tag to the end tag.
Everything should go inside of your html element.
Note: Tags should ALWAYS be lowercase.
Nesting Elements
Nesting is where an element contains another element.
<html>
<head>
<title>My First Web Page</title>
</head>
<body>
<img src="https://coursework.vschool.io/content/images/2015/05/logo-wo-text-2.png">
</body>
</html>
<head></head>
and <body></body>
are always nested inside of <html></html>
The head and the body will never ever be nested inside of the other. If you think of a web page as a person with a head and a body, then it only makes sense for the body to be connected to the head, but you can't fit the body into the head, nor the head into the body.
Aside from that rule, you can nest things as much as you'd like. Empty elements can't contain any other elements though, so be careful with those. It makes sense if you think about it though, because why would you want to put something inside of an image or a line break element?
Too often when elements are nested inside of each other I see them look messy.
<html>
<head>
<title></title>
</head>
<body>
<img src="https://coursework.vschool.io/content/images/2015/05/logo-wo-text-2.png">
</body>
</html>
The computer might treat this as it would pretty code, but as a developer you will have a very difficult time reading this code especially since html files are going to contain WAY more than just 5 elements.
There is no excuse for ugly code, it slows you down, it hinders other developers who are fixing or using your code, and it makes you look super incompetent. Instead you will always put a consistent amount of spaces on each line--most developers use 1 tab space to symbolize one level of nesting. If you nest something inside of an element that is already nested inside of another element then you must put two tab spaces.
HTML5
The new HTML5 elements can be found here.
<!DOCTYPE html>
Be sure to add this to the top of all of your HTML files.
From the W3 editors guide:
DOCTYPEs are required for legacy reasons. When omitted, browsers tend to use a different rendering mode that is incompatible with some specifications. Including the DOCTYPE in a document ensures that the browser makes a best-effort attempt at following the relevant specifications.'
Cool new media elements:
<audio>
- Defines sound or music content<embed>
- Defines containers for external applications (like plug-ins)<source>
- Defines sources for<video>
and<audio>
<track>
- Defines tracks for<video>
and<audio>
<video>
- Defines video or movie content