Debugging
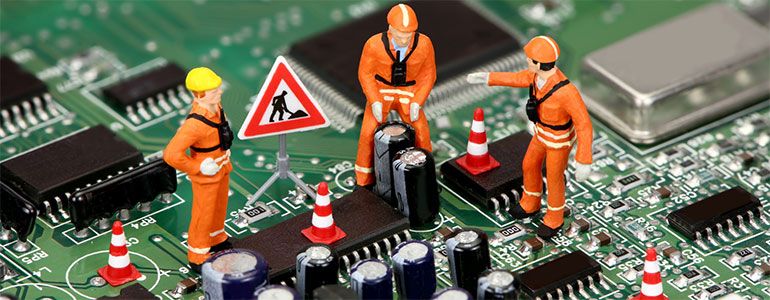
If you want to jump straight to the debugging checklist, click here.
Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.
- Brian Kernighan and P.J. Plauger, The Elements of Programming Style
The skill of debugging is an extraordinarily important one to develop as a programmer. No matter how good your code is or how skilled of a programmer you become, you will always spend a majority of your time fixing bugs in your (or someone else's) code.
What is debugging? What are bugs?
Bugs are, simply put, flaws in your program. Sometimes they are immediately apparent, sometimes they can remain in a system for years without being caught. Sometimes, bugs are simply programmer errors such as spelling mistakes or missing curly braces. Often, however, bugs only surface when someone uses your program in a way that you, the programmer, hadn't anticipated.
Debugging is an art AND a science.
The computer does exactly what you tell it to. End of story. It's science. You'll be humbled time and again if you let yourself believe otherwise. When "the program isn't doing what you told it to do", it's almost a certainty that you've made a mistake.
If the computer runs on science, and if programming can be considered an art, then the process of debugging is both an art and a science. Depending on the type of bug you're encountering, you will likely have to put on your think-like-a-computer hat AND your think-like-my-grandmother hat.
Debugging is a problem-solving process
Since debugging makes up such a significant portion of our time as programmers, there are tons of tools and techniques at your disposal you can use to find and squash the bugs you (or someone else) has written into the code.
This is one of the major places where junior developers are easy to distinguish from mid-level or senior developers. Junior developers tend to follow the following problem-solving strategy:
This guess-and-check method takes a long time and can clutter up or even damage the code that already works, and oftentimes doesn't lead to a better understanding of what the problem was in the first place.
The following diagrams represents how a mid or senior developer solves a problem:
The process generally consists of having a clear understanding of what the goal is, understanding the problem to the fullest extent possible, and methodically testing the different options available until the problem is solved. This process of problem solving typically not only leads to fixing the original bug, but oftentimes to the betterment of the entire code base in general.
Debugging checklist
There are a number of things you can do to be more methodical in how to go about squashing bugs. The following is definitely an incomplete list, but should be helpful in understanding what tools and tricks you have at your disposal as a programmer to be more methodical in fixing the bugs you encounter and create along the way.
Web development
- Check if your text editor is complaining about any syntactical problems with your code. Most modern editors will have code "linters" that check your syntax as you type.
- Make sure you variable names are good and that your indentation and spacing follows convention. It'll be easier to figure out what code is the issue this way.
- Check for spelling inconsistencies in your variable and function names. Sometimes linters won't catch when a variable is inconsistent from use to use.
- Remember, capitalization matters!
document.getElementByID()
isn't a real JavaScript function, whereasdocument.getElementById()
is.
- Remember, capitalization matters!
- Right-click & inspect element on the page with the problem
- Check the Console tab for any errors
- Check the Network tab to see if a request is failing or a resource isn't downloading correctly.
- JavaScript not reacting the way you expected? Check the Sources tab to set watch expressions for any JavaScript variables and place breakpoints in your JavaScript code to make sure your functions are getting run correctly. In this tab, you can also easily see which of your files were actually read into the browsers memory.
- In a NodeJS application, use the debugger tools built into Atom, VS Code, Webstorm, etc to check what your code is actually doing.
console.log()
statements inside the functions you're expecting to be run and print to the console the value of the variable at the current point in the program, or simply print a string of text so you know that code is being executed. This is useful when there's not an easy way to tell if your functions are being executed or not.- Remember to remove these once you know your function is working correctly! Don't leave useless console logging statements in your code.
- CSS styles not being applied? Check to make sure you linked it up in the index HTML page, and that the path is correct.