Containers Components
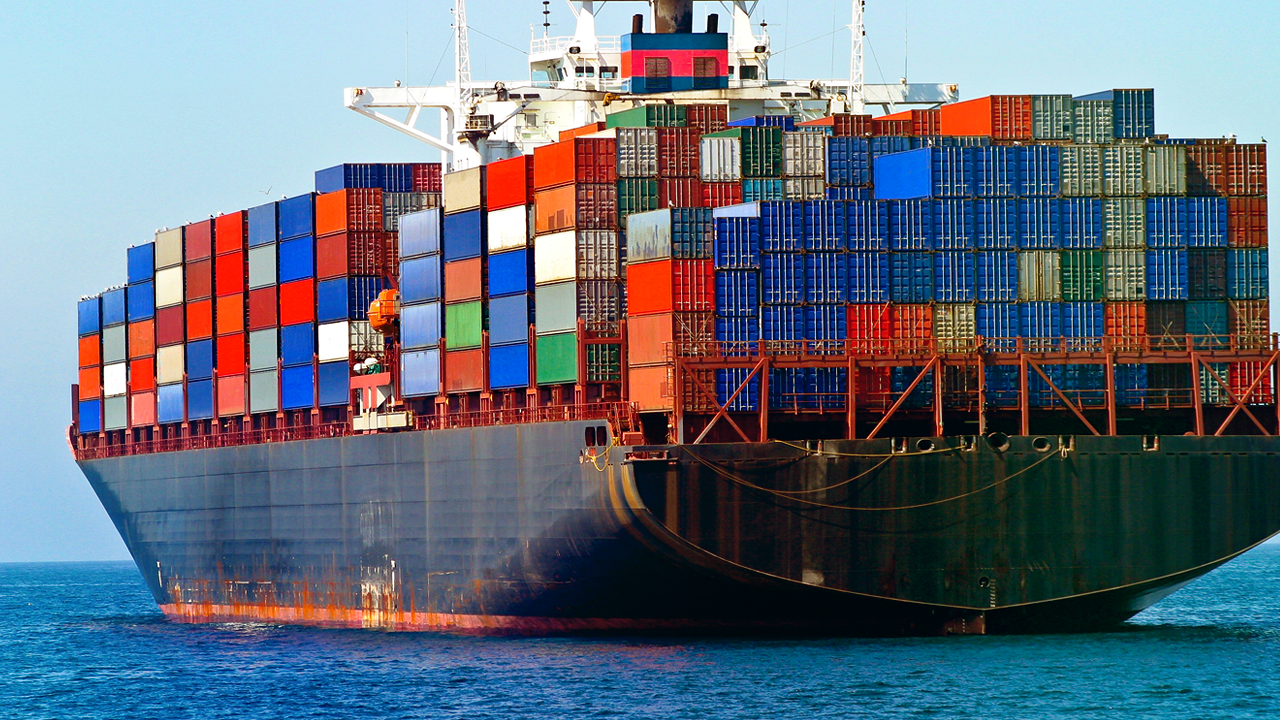
There are many ways to organize your React app, so much of it is learning popular, tried and true patterns and principles.
Maybe the most important of these is using Container Components. Container Components contain Presentation Components.
Basically, container(Smart or Stateful) components and components(Dumb Component or presentational component) are react components.
A container component is a react component that has direct connection with state and also called a 'smart component'
The Presentational Component is simple component that only renders the DOM elements to the screen. They are also called Dumb components
Container (Smart or Stateful) Components
- Are concerned with how things work.
- May contain both presentational and container components inside but usually don’t have any DOM markup of their own except for some wrapping divs, and never have any styles.
- Provide the data and behavior to presentational or other container components.
- Call Flux or Redux actions and provide these as callbacks to the presentational components.
- Are often stateful, as they tend to serve as data sources.
- Are usually generated using higher order components such as
connect()
from React Redux,createContainer()
from Relay, orContainer.create()
from Flux Utils, rather than written by hand.
Component(Presentational, Dumb or stateless) component
- Are concerned with how things look.
- May contain both presentational and container components inside, and usually have some DOM markup and styles of their own.
- Often allow containment via
this.props.children
andHave no dependencies on the rest of the app, such as Flux or Redux actions or stores. - Don’t specify how the data is loaded or mutated.
- Receive data and callbacks exclusively via props.
- Rarely have their own state (when they do, it’s UI state rather than data).
Benefits of This Approach
- Better separation of concerns. You understand your app and your UI better by writing components this way.
- Better reusability. You can use the same presentational component with completely different state sources, and turn those into separate container components that can be further reused.
- Presentational components are essentially your app’s “palette”. You can put them on a single page and let the designer tweak all their variations without touching the app’s logic. You can run screenshot regression tests on that page.
This forces you to extract “layout components” such as Sidebar, Page, ContextMenu and use this.props.children instead of duplicating the same markup and layout in several container components.