Components In React
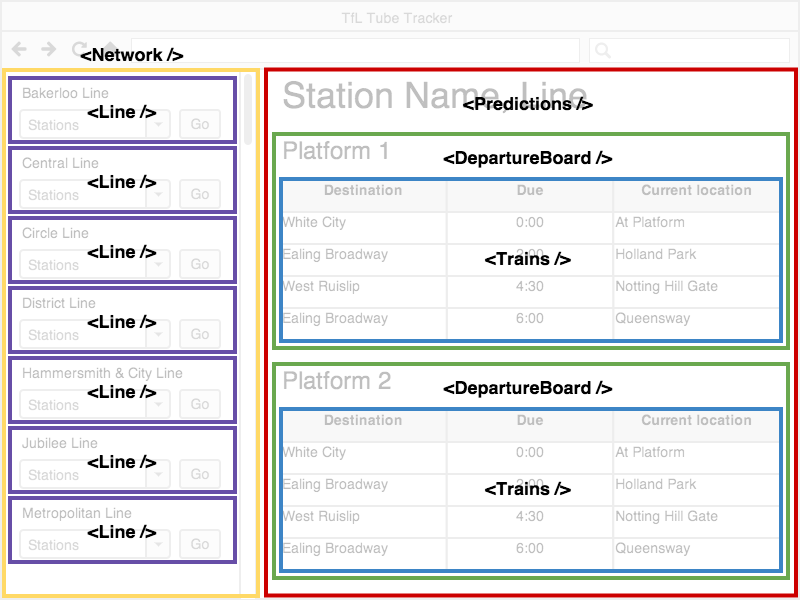
What is a component?
A component is a part or element of a larger whole. In React a component is just that, a part of a website. Examples of this include Navbar, Header, Footer, in fact, we could think of a website as being completely made of components.
In React, components let you split the UI into independent, reusable pieces, and think about each piece in isolation. You can think of them like functions that show an element onto the screen when called.
Why use them?
Breaking your UI into pieces holds a couple of advantages. First off by breaking your code into smaller pieces, you make debugging your code a lot easier. It also makes your components modular so you can take those components and move them to other projects.
How to make a component
// ./components/myComponent/MyComponent.js
import React from "react";
//Make a new class and extend React.Component to use reacts component code
function MyComponent() {
//inside it will return some JSX
return (
<h1>
Hello World
</h1>
)
}
//export your component so it can be used in your app
export default MyComponent;
Using a component
You can now import this component and use it as many times as you like. To use it simply put the name of the component surrounded by a less than (<) and a greater than (>) symbol.
import React from "react"
import MyComponent from "./components/myComponent/MyComponent.js";
function App() {
return (
<div>
{/* How you call a component */}
<MyComponent />
</div>
)
}
export default App;