Building a Simple Web Game (Approaching a larger project)
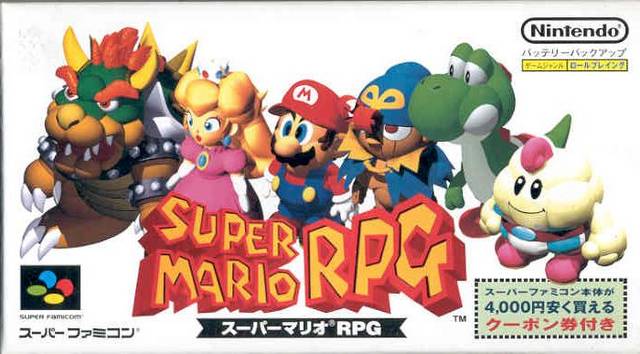
This article is going to explain how to approach building a larger project as well as how to build a simple RPG web game
Wireframing
It is always good to wireframe your website on paper (or in Photoshop/etc) before you start laying things out in code.
Just draw up a few wireframes of the different screens/states to give yourself an idea of how it might work.
HTML & Bootstrap
The next step is to layout HTML. Include your buttons, images, etc. It is ok if they are all static right now. You will also want to lay out everything using the Bootstrap grid system.
This is the time to make sure you are setting the proper CSS id
attributes on their corresponding elements. So if you have an img
for the player you might set it's id to <img id="player-img" />
etc.
Make sure that you continually check that your app looks great on smaller screen sizes. Every time you finish some layout in HTML check it on a smaller screen!
The Code
You will want to put your code in one or more .js
files.
Data Model
The first step is create your data model objects. Meaning if you have things in your app that need to be logically grouped together, now is the time to do this (before writing functions and game logic).
So this is where I might define my Player
and Enemy
objects.
var Player = function(name) {
this.name = name;
this.hp = 100;
this.attackPwr = 15;
};
//etc, etc
Functions/Logic
After your data model is defined start creating the functions needed to run the game. Write handlers for DOM events. For instance, if you have a Start Game
button in your HTML you might want an even handler such as:
var startGameBtnClicked = function(event) {
//Create a new player
//Create a new enemy
//Call some other functions to get game started?
}
CSS/Beautification
You will probably want to focus on CSS/making your site pretty last!