Angular Core Overview
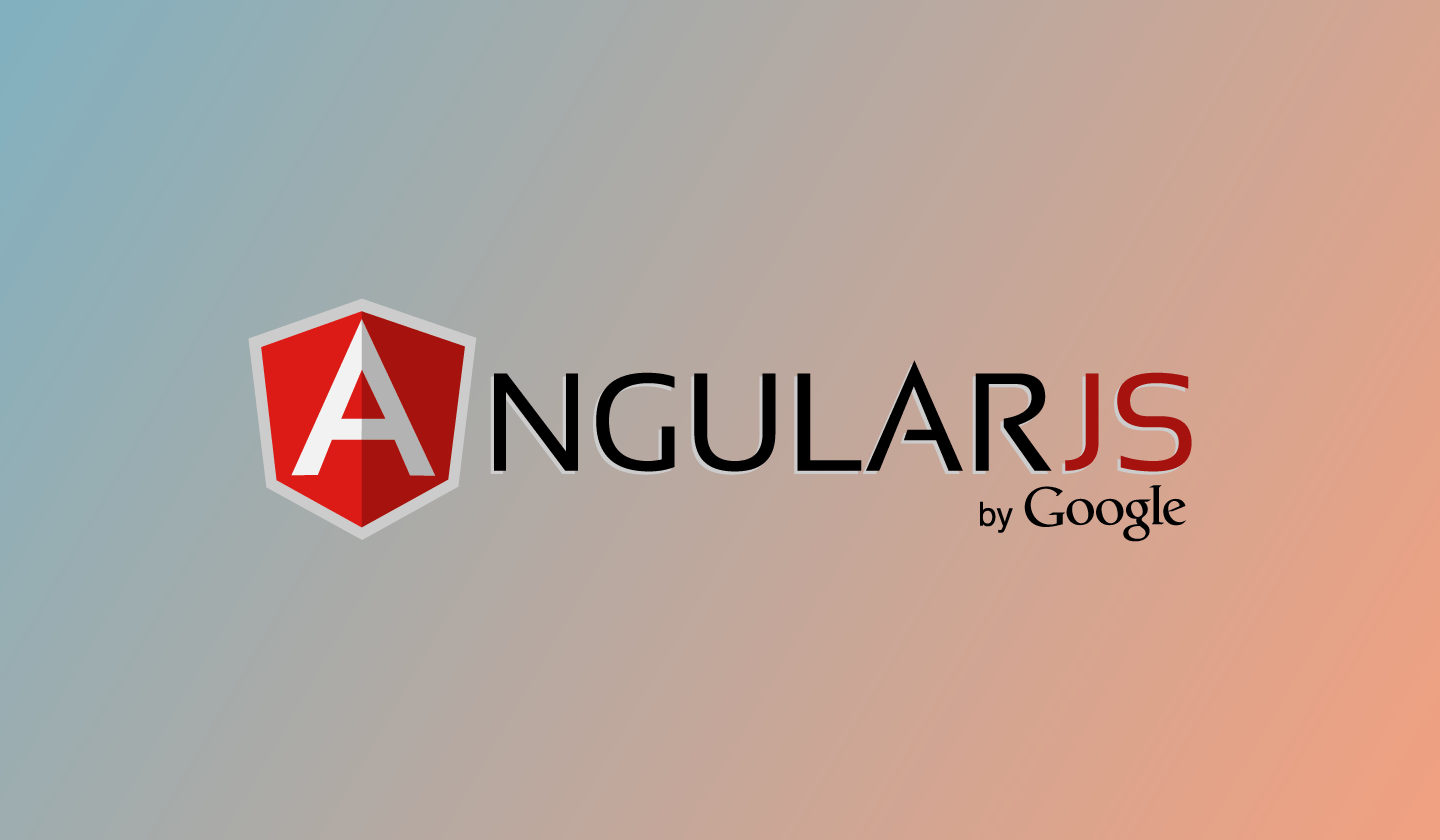
Angular is a large, complex framework. However, its full power can be used with minimal effort.
Installation
Install Angular by including it in your html:
<html ng-app="learningAngular">
<head>
<link rel="stylesheet" href="//maxcdn.bootstrapcdn.com/bootswatch/3.3.4/cerulean/bootstrap.min.css">
</head>
<body>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/angular.min.js"></script>
</body>
</html>
## Modules
Angular apps can have one or more modules. Modules are singletons and all Angular controllers and services must belong to a module. Modules can also include other modules as dependencies.
When declaring a module, you include the module name as the first parameter and enter an array of dependencies as the second parameter.
A simple module declaration:
var app = angular.module('learningAngular',[]);
To use your module you must include it in your html using the ng-app directive.
<html ng-app="learningAngular">
<head>
<link rel="stylesheet" href="//maxcdn.bootstrapcdn.com/bootswatch/3.3.4/cerulean/bootstrap.min.css">
</head>
<body>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/angular.min.js"></script>
</body>
</html>
##Controllers Controllers are the meat of Angular. They play a key role in the MVC paradigm. A module can have one or more controllers. A controller can be declared like this:
var app = angular.module('learningAngular',[]);
app.controller('mainCtrl',['$scope', function($scope) {
}]);
A controller consists of a name as the first parameter, and an array as the second parameter if you plan on minifying your javascript. Basically in this minified style you declare the needed dependencies as a string, and then a function as the second element of the array that has those dependencies as arguments. The non-minified version is simpler:
app.controller('mainCtrl', function($scope) {
});
To use a controller, you can insert it into your html like this:
<html ng-app="learningAngular">
<head>
<link rel="stylesheet" href="//maxcdn.bootstrapcdn.com/bootswatch/3.3.4/cerulean/bootstrap.min.css">
</head>
<body ng-controller="mainCtrl">
<!-- Everything inside of the body has access to this controllers $scope variable -->
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/angular.min.js"></script>
</body>
</html>
##$scope
$scope is the glue that binds your html to your controllers. Your controllers perform the logic and equations, and then pass the data through the $scope to your html and vice versa.
Every controller has a $scope. If you include ng-controllers within other ng-controllers, the innter controllers will have access to it's own scope, as well as the scope of the parent controller.
Here is an example of how to make data available to your html via $scope:
var app = angular.module('learningAngular',[]);
app.controller('mainCtrl',['$scope', function($scope) {
$scope.person = {
firstName: "John",
lastName: "Smith"
};
$scope.getFullName = function() {
return $scope.person.firstName + ' ' + $scope.person.lastName;
}
}]);
This is how you might access your $scope data:
<html ng-app="learningAngular">
<head>
<link rel="stylesheet" href="//maxcdn.bootstrapcdn.com/bootswatch/3.3.4/cerulean/bootstrap.min.css">
</head>
<body ng-controller="mainCtrl">
<!-- Everything inside of the body has access to this controllers $scope variable -->
<div>
<h3>{{person.firstName}} {{person.lastName}}</h3>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/angular.min.js"></script>
<script src="main.js"></script>
</body>
</html>
Notice the double curly braces:
{{person.firstName}}
Those curly braces will grab $scope data from your controller. We could also have done:
<body ng-controller="mainCtrl">
<!-- Everything inside of the body has access to this controllers $scope variable -->
<div>
<h3>{{getFullName()}}</h3>
</div>
</body>
In this case we instead call our $scope function getFullName(). You are in essence writing Javascript between the double curly braces.
The end result:
ng-repeat
When you place an ng-repeat attribute on an HTML element it will repeat whatever HTML you have written and all of its sub-elements until the expression within the ng-repeat attribute is fulfilled.
HTML:
<-- this div will repeat for each iteration of the array todos -->
<div ng-repeat="item in todos">
<h1> {{item.name}}</h1>
<h3> {{item.price}}</h3>
</div>
Angular Javascript:
$scope.todos = [
{
name: "Bread",
price: "$2.00"
},
{
name: "Milk",
price: "$2.99"
},
{
name: "Protein",
price: "$14.50"
}
]
Services
Angular services are singletons. They can be used module wide or application wide. Services are where you should store data that you don't want to lose between screens and different controllers.
They are also great for modularizing your code. You might create a service that performs network/http requests. You might have a service that stores global data for your application, or a service where you can access constants to prevent code duplication.
This syntax can be written as follows:
var app = angular.module('learningAngular');
app.service('dataService', function() {
this.personLoggedIn = undefined;
});
We put this code in a separate file this time - services can live in the same script where its parent module is declared or they can be in other script files. If you put a service in another script file, you have to grab a reference to your module as we did above. This time you don't add any dependencies since you aren't creating the module, but just referencing it.
To use your service in your controller you'll need to include it as a dependency as follows:
var app = angular.module('learningAngular',[]);
app.controller('mainCtrl', function($scope, dataService) {
$scope.person = {
firstName: "John",
lastName: "Smith"
};
$scope.getFullName = function() {
return $scope.person.firstName + ' ' + $scope.person.lastName;
}
dataService.personLoggedIn = $scope.person;
});
##ng-model
Angular's ng-model is used for two-way data binding and is typically used on form elements. We'll add an html input and set an ng-model of title. We'll also change the data in the double curly braces to be title.
<html ng-app="learningAngular">
<head>
<link rel="stylesheet" href="//maxcdn.bootstrapcdn.com/bootswatch/3.3.4/cerulean/bootstrap.min.css">
</head>
<body ng-controller="mainCtrl">
<!-- Everything inside of the body has access to this controllers $scope variable -->
<div>
<h3>{{title}}</h3>
<input type="text" ng-model="title" />
</div>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/angular.min.js"></script>
<script src="main.js"></script>
<script src="dataservice.js"></script>
</body>
</html>
Here are the changes to our controller:
app.controller('mainCtrl', function($scope, dataService) {
$scope.person = {
firstName: "John",
lastName: "Smith"
};
$scope.getFullName = function() {
return $scope.person.firstName + ' ' + $scope.person.lastName;
}
$scope.title = "TEST"
dataService.personLoggedIn = $scope.person;
});
Now if you load your web page and start typing in the box - your h3 element will reflect whatever you are typing in because we are now binding that input box to the title, and the title is being displayed in the h3.