Yoda Speak
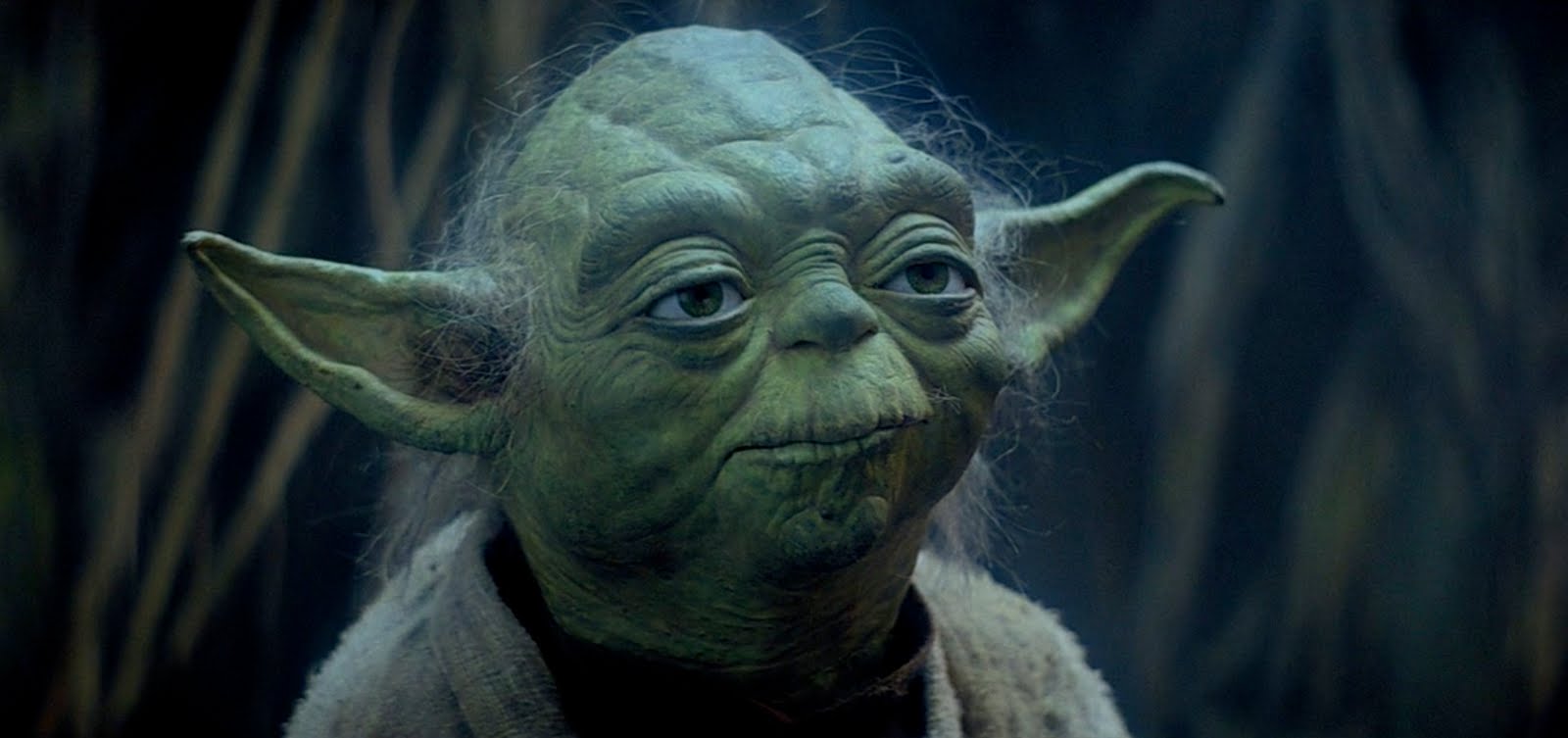
This is an $http exercise.
You have been commissioned as Yoda's official translator, and to make your job easier you want to create a site that translates normal speech into Yoda Speak. Luckily, a Yoda Speak API already exists so all you have to do is connect with the API using Angular.
The app is very simple. This is how it looks:
There is a text box in which the user inputs a sentence. When they press the translate button, a request is sent to the API to get the translated text. The translated text is then show to the user.
Sample Request
Method: GET
URL: https://yoda.p.mashape.com/yoda?sentence=You will learn how to speak like Yoda someday
Header: "X-Mashape-Key": "ydoXoQrHFfmsh6N8IE3C6r1Osz8ep1MAkh2jsnZZX99BCTLHAm"
How to include headers in an Angular $http
request
The $http.get()
method takes an optional second parameter (the first required one being the URL for the request). That second parameter is a configuration object that allows you to add additional information about the request, such as the query parameters or any additional headers to the request.)
The API that translates your text into Yoda Speak text requires an API key in order to work. You need to add that to the headers of the request:
var config = {
headers: {
'X-Mashape-Key': 'ydoXoQrHFfmsh6N8IE3C6r1Osz8ep1MAkh2jsnZZX99BCTLHAm'
}
};
// You don't NEED to define it in a separate variable, but it makes it easier to read and edit.
$http.get("your-url-here", config)...
Response
Learn how to speak like yoda someday, you will. Herh herh herh.
(Try this out in Postman before writing it in Angular. The API is pretty slow so you may have to wait a few seconds.)