Intro to Mongoose
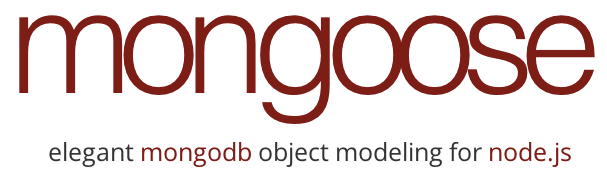
Mongoose is a library for connecting to MongoDB and running queries from a Node.js app. Installation is trivial: npm install --save mongoose
.
Although documents in a MongoDB collection do not have to be homogeneous (they may have different schemas), Mongoose enforces document schemas to make the collection more uniform (and they help a lot). Here is how you connect to MongoDB with Mongoose:
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/db-name',{useNewUrlParser: true})
.then(()=> console.log("Connected to MongoDB"))
.catch(err => console.error(err));
You may replace db-name
with whatever your database is called. localhost
implies that MongoDB is running on your local machine, but it is possible to use a remote database service such as MongoLab.
Once you have a connection to MongoDB, it's time to make some schemas. Each schema is associated with a MongoDB collection. Here is how we would write our friend schema from yesterday:
const Schema = mongoose.Schema;
const friendSchema = new Schema({
name: String,
age: Number,
interests: [String],
friends: [String]
});
Right now this schema is nothing more than a template of how each document in the friends
collection will look. To be able to add new friends, and update and delete them (etc.), we must register the schema with mongoose and make a model:
const Friend = mongoose.model('Friend', friendSchema);
Now that we have a data model to work with, we can perform all the CRUD operations from within Node.
Here's what a create would look like:
const myFriend = new Friend({
name: 'JD',
age: 24,
interests: ['Music', 'Food'],
friends: []
});
myFriend.save((err, new_friend) => {
console.log(new_friend);
});
Here's a read:
Friend.find({age: 24}, (err, friends) => {
console.log(friends);
});
This will return a maximum of one document:
Friend.findOne({age: 24},(err, friend) => {
console.log(friend);
});
Here's how you update a document:
Friend.findOne({name: 'JD'}, (err, friend) => {
friend.age++;
friend.save((err, new_friend) => {
console.log(new_friend);
});
});
And finally, to remove a document:
Friend.findOne({name: 'JD'}, (err, friend) => {
friend.remove(err => {
console.log('JD is gone');
});
});
Some important notes:
err
is only set if there is an error with the database, not if nothing is found- For the
findOne
function, if nothing is found the data parameter isnull
- If you need to access a particular model (called
Friend
for example), just usemongoose.model('Friend')