jQuery
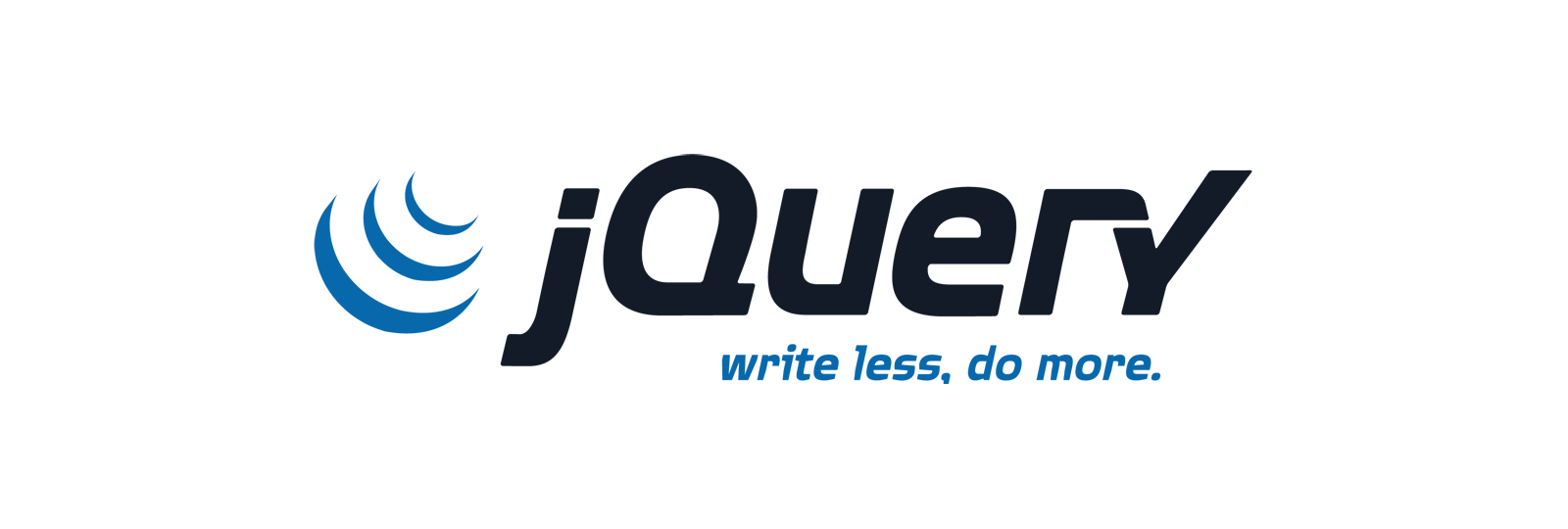
Getting Started With jQuery
It's a JavaScript library that greatly simplifies JavaScript programming, and the best part is, it's easy to learn.
The MEAN stack includes AngularJS which will largely remove the need to use jQuery, however jQuery is included as part of Angular and you will use it from time to time within your code.
JQuery is an extremely useful skill set and will be important to you throughout your career, even if you specialize in AngularJS or another framework.
Installation
Installation is easy just include jQuery in a script tag on your HTML page the same way you'd do for any other external script file.
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.4/jquery.min.js"></script>
Put the code above at the bottom of the <body></body>
element of your page, above your own personal javascript files.
See other options for installation on the jQuery website.
Other Notes Before You Start
If you're going to write jQuery code you're going to want to do so in a script. Create your script, naming it whatever you'd like, and then link to it the same way you did the jQuery file. Of course you will need to put in the appropriate filepath from your html file to your js file.
<script src="myScript.js"></script>
It's worth noting if you're new to jQuery that even though you're using jQuery code, you can still use regular JavaScript code in the same file, and you'll still use the .js extension when you save the script file.
Selectors
The most basic concept of jQuery is to "select some elements and do something with them." jQuery supports most CSS3 selectors, as well as some non-standard selectors. For a complete selector reference, visit the Selectors documentation on api.jquery.com .
Selecting Elements by ID
$( "#myId" ); // Note IDs must be unique per page.
Selecting Elements by Class Name
$( ".myClass" );
Selecting Elements by Attribute
$( "input[name='first_name']" ); // Beware, this can be very slow in older browsers
Selecting Elements by Compound CSS Selector
$( "#contents ul.people li" );
Pseudo-Selectors
$( "a.external:first" );
$( "tr:odd" );
// Select all input-like elements in a form (more on this below).
$( "#myForm :input" );
$( "div:visible" );
// All except the first three divs.
$( "div:gt(2)" );
// All currently animated divs.
$( "div:animated" );
When using the :visible and :hidden pseudo-selectors, jQuery tests the actual visibility of the element, not its CSS visibility or display properties. jQuery looks to see if the element's physical height and width on the page are both greater than zero.
However, this test doesn't work with
Elements that have not been added to the DOM will always be considered hidden, even if the CSS that would affect them would render them visible. See the Manipulating Elements section to learn how to create and add elements to the DOM.
The best way to determine if there are any elements is to test the selection's .length property, which tells you how many elements were selected. If the answer is 0, the .length
property will evaluate to false when used as a boolean value:
// Testing whether a selection contains elements.
if ( $( "div.foo" ).length ) {
...
}
jQuery doesn't cache elements for you. If you've made a selection that you might need to make again, you should save the selection in a variable rather than making the selection repeatedly.
var divs = $( "div" );
Once the selection is stored in a variable, you can call jQuery methods on the variable just like you would have called them on the original selection.
Sometimes the selection contains more than what you're after. jQuery offers several methods for refining and filtering selections.
// Refining selections.
$( "div.foo" ).has( "p" ); // div.foo elements that contain <p> tags
$( "h1" ).not( ".bar" ); // h1 elements that don't have a class of bar
$( "ul li" ).filter( ".current" ); // unordered list items with class of current
$( "ul li" ).first(); // just the first unordered list item
$( "ul li" ).eq( 5 ); // the sixth
jQuery offers several pseudo-selectors that help find elements in forms. These are especially helpful because it can be difficult to distinguish between form elements based on their state or type using standard CSS selectors.
Not to be confused with :checkbox
, :checked
targets checked checkboxes, but keep in mind that this selector works also for checked radio buttons, and
$( "form :checked" );
$( "form :disabled" );
$( "form :enabled" );
$( "form :input" );
$( "form :selected" );
for selecting inputs of a specific type you can use the following: :password
:reset
:radio
:text
:submit
:checkbox
:button
:image
:file
Traversing
Once you've made an initial selection with jQuery, you can traverse deeper into what was just selected. Traversing can be broken down into three basic parts: parents, children, and siblings. jQuery has an abundance of easy-to-use methods for all these parts. Notice that each of these methods can optionally be passed string selectors, and some can also take another jQuery object in order to filter your selection down. Pay attention and refer to the API documentation on traversing to know what variation of arguments you have available.
The methods for finding the parents from a selection include .parent()
, .parents()
, .parentsUntil()
, and .closest()
.
<div class="grandparent">
<div class="parent">
<div class="child">
<span class="subchild"></span>
</div>
</div>
<div class="surrogateParent1"></div>
<div class="surrogateParent2"></div>
</div>
// Selecting an element's direct parent:
// returns [ div.child ]
$( "span.subchild" ).parent();
// Selecting all the parents of an element that match a given selector:
// returns [ div.parent ]
$( "span.subchild" ).parents( "div.parent" );
// returns [ div.child, div.parent, div.grandparent ]
$( "span.subchild" ).parents();
// Selecting all the parents of an element up to, but *not including* the selector:
// returns [ div.child, div.parent ]
$( "span.subchild" ).parentsUntil( "div.grandparent" );
// Selecting the closest parent, note that only one parent will be selected
// and that the initial element itself is included in the search:
// returns [ div.child ]
$( "span.subchild" ).closest( "div" );
// returns [ div.child ] as the selector is also included in the search:
$( "div.child" ).closest( "div" );
The methods for finding child elements from a selection include .children()
and .find()
. The difference between these methods lies in how far into the child structure the selection is made. .children()
only operates on direct child nodes, while .find()
can traverse recursively into children, children of those children, and so on.
// Selecting an element's direct children:
// returns [ div.parent, div.surrogateParent1, div.surrogateParent2 ]
$( "div.grandparent" ).children( "div" );
// Finding all elements within a selection that match the selector:
// returns [ div.child, div.parent, div.surrogateParent1, div.surrogateParent2 ]
$( "div.grandparent" ).find( "div" );
The rest of the traversal methods within jQuery all deal with finding sibling selections. There are a few basic methods as far as the direction of traversal is concerned. You can find previous elements with .prev()
, next elements with .next()
, and both with .siblings()
. There are also a few other methods that build onto these basic methods: .nextAll()
, .nextUntil()
, .prevAll()
and .prevUntil()
.
// Selecting a next sibling of the selectors:
// returns [ div.surrogateParent1 ]
$( "div.parent" ).next();
// Selecting a prev sibling of the selectors:
// returns [] as No sibling exists before div.parent
$( "div.parent" ).prev();
// Selecting all the next siblings of the selector:
// returns [ div.surrogateParent1, div.surrogateParent2 ]
$( "div.parent" ).nextAll();
// returns [ div.surrogateParent1 ]
$( "div.parent" ).nextAll().first();
// returns [ div.surrogateParent2 ]
$( "div.parent" ).nextAll().last();
// Selecting all the previous siblings of the selector:
// returns [ div.surrogateParent1, div.parent ]
$( "div.surrogateParent2" ).prevAll();
// returns [ div.surrogateParent1 ]
$( "div.surrogateParent2" ).prevAll().first();
// returns [ div.parent ]
$( "div.surrogateParent2" ).prevAll().last();
Use .siblings()
to select all siblings.
// Selecting an element's siblings in both directions that matches the given selector:
// returns [ div.surrogateParent1, div.surrogateParent2 ]
$( "div.parent" ).siblings();
// returns [ div.parent, div.surrogateParent2 ]
$( "div.surrogateParent1" ).siblings();
Be cautious when traversing long distances in documents – complex traversal makes it imperative that the document's structure remain the same, which is difficult to guarantee even if you're the one creating the whole application from server to client. One- or two-step traversal is fine, but it's best to avoid traversals that go from one container to another.
Events
jQuery makes it straightforward to set up event-driven responses on page elements. These events are often triggered by the end user's interaction with the page, such as when text is entered into a form element or the mouse pointer is moved. In some cases, such as the page load and unload events, the browser itself will trigger the event.
jQuery offers convenience methods for most native browser events. These methods — including .click()
, .focus()
, .blur()
, .change()
, etc. — are shorthand for jQuery's .on()
method. The on method is useful for binding the same handler function to multiple events, when you want to provide data to the event handler, when you are working with custom events, or when you want to pass an object of multiple events and handlers.
Event setup using a convenience method
$( "p" ).click(function() {
console.log( "You clicked a paragraph!" );
});
Equivalent event setup using the .on()
method
$( "p" ).on( "click", function() {
console.log( "click" );
});
To remove an event listener, you use the .off()
method and pass in the event type to off. If you attached a named function to the event, then you can isolate the event tear down to just that named function by passing it as the second argument.
// Tearing down all click handlers on a selection
$( "p" ).off( "click" );
Quite often elements in your application will be bound to multiple events. If multiple events are to share the same handling function, you can provide the event types as a space-separated list to .on():
// Multiple events, same handler
$( "input" ).on("click change", // Bind handlers for multiple events
function() {
console.log( "An input was clicked or changed!" );
}
);
When each event has its own handler, you can pass an object into .on()
with one or more key/value pairs, with the key being the event name and the value being the function to handle the event.
// Binding multiple events with different handlers
$( "p" ).on({
"click": function() { console.log( "clicked!" ); },
"mouseover": function() { console.log( "hovered!" ); }
});
Sometimes you need a particular handler to run only once — after that, you may want no handler to run, or you may want a different handler to run. jQuery provides the .one()
method for this purpose.
// Switching handlers using the `.one()` method
$( "p" ).one( "click", firstClick );
function firstClick() {
console.log("You just clicked this for the first time!");
}
// Now set up the new handler for subsequent clicks;
// omit this step if no further click responses are needed
$(this).click(function () {
console.log("You have clicked this before!");
});
Note that in the code snippet above, the firstClick function will be executed for the first click on each paragraph element rather than the function being removed from all paragraphs when any paragraph is clicked for the first time.
.one()
can also be used to bind multiple events:
// Using .one() to bind several events
$( "input[id]" ).one( "focus mouseover keydown", firstEvent);
function firstEvent(eventObject) {
console.log("A " + eventObject.type + " event occurred for the first time on the input with id " + this.id);
}
In this case, the firstEvent function will be executed once for each event. For the snippet above, this means that once an input element gains focus, the handler function will still execute for the first keydown event on that element.
The this
Keyword
When jQuery calls a handler, the this keyword is a reference to the element where the event is being delivered; for directly bound events this is the element where the event was attached and for delegated events this is an element matching selector. (Note that this may not be equal to event.target if the event has bubbled from a descendant element.) To create a jQuery object from the element so that it can be used with jQuery methods, use $( this )
.
Some Useful jQuery Methods
.html()
- Get the HTML contents of the first element in the set of matched elements or set the HTML contents of every matched element.
$( "div.demo-container" ).html();
One can also set the HTML contents of each element in the set of matched elements. Consider the following HTML:
<div class="demo-container">
<div class="demo-box">Demonstration Box</div>
</div>
The content of <div class="demo-container">
can be set like this:
$( "div.demo-container" )
.html( "<p>All new content. <em>You bet!</em></p>" );
That line of code will replace everything inside <div class="demo-container">
.
.append()
- Insert content, specified by the parameter, to the end of each element in the set of matched elements. Inserts the specified content as the last child of each element in the jQuery collection.
$( ".inner" ).append( "<p>Test</p>" );
.prepend()
- Insert content, specified by the parameter, to the beginning of each element in the set of matched elements. Inserts the specified content as the first child of each element in the jQuery collection.
$( ".inner" ).prepend( "<p>Test</p>" );
.css()
- Get the value of a computed style property for the first element in the set of matched elements or set one or more CSS properties for every matched element.
$('#elementID').css( "background-color" );
Also can set one or more CSS properties for the set of matched elements.
$('#elementID').css("color", "#fff");
$('#elementID').css({ "background-color": "#ffe", "border-left": "5px solid #ccc" });
.hasClass()
- Determine whether any of the matched elements are assigned the given class.
<div id="mydiv" class="foo bar"></div>
$( "#mydiv" ).hasClass( "foo" );
.addClass()
- Adds the specified class(es) to each element in the set of matched elements. It's important to note that this method does not replace a class. It simply adds the class, appending it to any which may already be assigned to the elements.
More than one class may be added at a time, separated by a space, to the set of matched elements, like so:
$( "p" ).addClass( "myClass yourClass" );
.removeClass()
- Remove a single class, multiple classes, or all classes from each element in the set of matched elements. If a class name is included as a parameter, then only that class will be removed from the set of matched elements. If no class names are specified in the parameter, all classes will be removed.
$( "p" ).removeClass( "myClass yourClass" )
This method is often used with .addClass() to switch elements' classes from one to another, like so:
$( "p" ).removeClass( "myClass noClass" ).addClass( "yourClass" );
.toggleClass()
- Add or remove one or more classes from each element in the set of matched elements, depending on either the class's presence or the value of the state argument.
<div class="tumble">Some text.</div>
$( "div.tumble" ).toggleClass( "bounce" )
This returns the following html:
<div class="tumble bounce">Some text.</div>
.width()
- The difference between .css(width)
and .width()
is that the latter returns a unit-less pixel value (for example, 400) while the former returns a value with units intact (for example, 400px). The .width()
method is recommended when an element's width needs to be used in a mathematical calculation.
// Returns width of browser viewport
$( window ).width();
// Returns width of HTML document
$( document ).width();
.height()
- The difference between .css( "height" )
and .height() is that the latter returns a unit-less pixel value (for example, 400) while the former returns a value with units intact (for example, 400px). The .height()
method is recommended when an element's height needs to be used in a mathematical calculation.
$( "p" ).height();
Can also set the CSS height of every matched element.
$( this ).height( 30 );
jQuery offers a variety of methods for obtaining and modifying dimension and position information about an element.
// Basic dimensions methods.
// Sets the width of all <h1> elements.
$( "h1" ).width( "50px" );
// Gets the width of the first <h1> element.
$( "h1" ).width();
// Sets the height of all <h1> elements.
$( "h1" ).height( "50px" );
// Gets the height of the first <h1> element.
$( "h1" ).height();
// Returns an object containing position information for
// the first <h1> relative to its "offset (positioned) parent".
$( "h1" ).position();
Ajax and Http Requests
Unfortunately, different browsers implement the Ajax API differently. Typically this meant that developers would have to account for all the different browsers to ensure that Ajax would work universally. Fortunately, jQuery provides Ajax support that abstracts away painful browser differences. It offers both a full-featured $.ajax()
method, and simple convenience methods such as $.get()
, $.getScript()
, $.getJSON()
, $.post()
, and $().load()
.
Most jQuery applications don't in fact use XML, despite the name "Ajax"; instead, they transport data as plain HTML or JSON (JavaScript Object Notation).
In general, Ajax does not work across domains. For instance, a webpage loaded from example1.com is unable to make an Ajax request to example2.com as it would violate the same origin policy. As a work around, JSONP (JSON with Padding) uses <script>
tags to load files containing arbitrary JavaScript content and JSON, from another domain. More recently browsers have implemented a technology called Cross-Origin Resource Sharing (CORS), that allows Ajax requests to different domains.
While jQuery does offer many Ajax-related convenience methods, the core $.ajax()
method is at the heart of all of them, and understanding it is imperative. We'll review it first, and then touch briefly on the convenience methods.
It's often considered good practice to use the $.ajax()
method over the jQuery provided convenience methods. As you'll see, it offers features that the convenience methods do not, and its syntax allows for the ease of readability.
$.ajax()
jQuery’s core $.ajax()
method is a powerful and straightforward way of creating Ajax requests. It takes a configuration object that contains all the instructions jQuery requires to complete the request. The $.ajax()
method is particularly valuable because it offers the ability to specify both success and failure callbacks. Also, its ability to take a configuration object that can be defined separately makes it easier to write reusable code.
// Using the core $.ajax() method
$.ajax({
// The URL for the request
url: "post.php",
// The data to send (will be converted to a query string)
data: {
id: 123
},
// Whether this is a POST or GET request
method: "GET",
// The type of data we expect back
dataType : "json",
// Code to run if the request succeeds;
// the response is passed to the function
success: function( json ) {
$( "<h1>" ).text( json.title ).appendTo( "body" );
$( "<div class=\"content\">").html( json.html ).appendTo( "body" );
},
// Code to run if the request fails; the raw request and
// status codes are passed to the function
error: function( xhr, status, errorThrown ) {
alert( "Sorry, there was a problem!" );
console.log( "Error: " + errorThrown );
console.log( "Status: " + status );
console.dir( xhr );
},
// Code to run regardless of success or failure
complete: function( xhr, status ) {
alert( "The request is complete!" );
}
});
The .load()
method is unique among jQuery’s Ajax methods in that it is called on a selection. The .load()
method fetches HTML from a URL, and uses the returned HTML to populate the selected element(s). In addition to providing a URL to the method, you can optionally provide a selector; jQuery will fetch only the matching content from the returned HTML.
// Using .load() to populate an element
$( "#newContent" ).load( "/foo.html" );
// Using .load() to populate an element based on a selector
$( "#newContent" ).load( "/foo.html #myDiv h1:first", function( html ) {
alert( "Content updated!" );
});
Plugins
A jQuery plugin is simply a new method that we use to extend jQuery's prototype object. By extending the prototype object you enable all jQuery objects to inherit any methods that you add. As established, whenever you call jQuery() you're creating a new jQuery object, with all of jQuery's methods inherited.
The idea of a plugin is to do something with a collection of elements. You could consider each method that comes with the jQuery core a plugin, like .fadeOut()
or .addClass()
.
You can make your own plugins and use them privately in your code or you can release them into the wild. There are thousands of jQuery plugins available online. You can also easily make your own.
Searching for a Plugin
One of the most celebrated aspects of jQuery is its extensive plugin ecosystem. From table sorting to form validation to autocompletion – if there's a need for it, chances are good that someone has written a plugin for it.
The quality of jQuery plugins varies widely. Many plugins are extensively tested and well-maintained, but others are hastily created and then ignored. More than a few fail to follow best practices. Some plugins, mainly jQuery UI, are maintained by the jQuery team. The quality of these plugins is as good as jQuery itself.
Another great resource for finding 3rd party plugins made with jQuery can be found at http://plugins.jquery.com/.
Creating your own Plugins
Sometimes you want to make a piece of functionality available throughout your code. For example, perhaps you want a single method you can call on a jQuery selection that performs a series of operations on the selection. In this case, you may want to write a plugin.
Read more about this subject at the Learn jQuery Website!