Hoisting With Functions
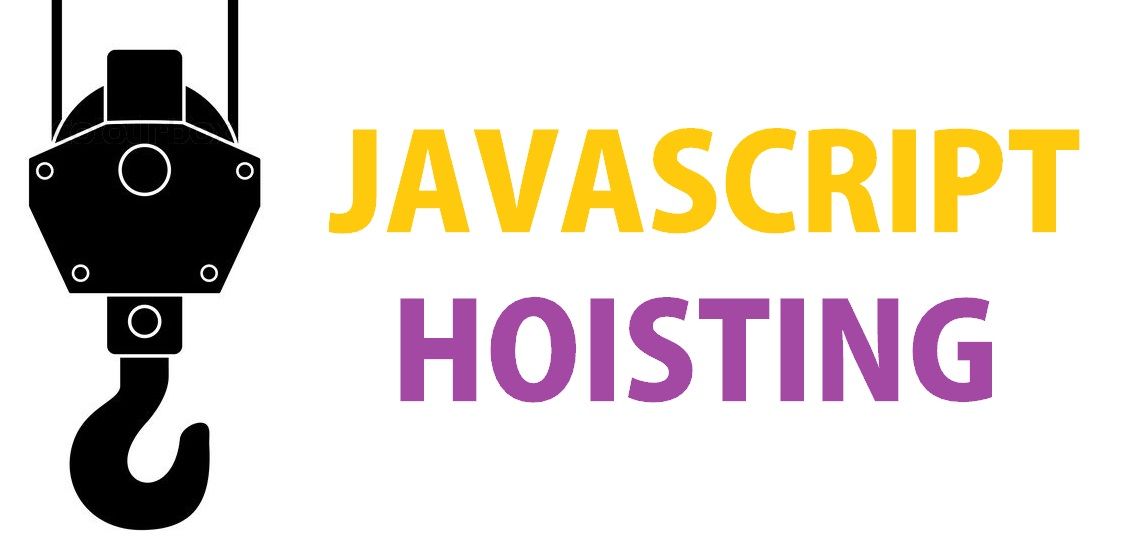
What is hoisting?
Hoisting is a general way of describing how JavaScript is read. Certain syntax of functions will, in a sense, cause the declared functions to be hoisted to the beginning of the code.
Before diving deeper into how hoisting works and what it means for us, let's take a quick look at function declarations and function expressions.
Function declaration is one way of defining a function, and differs from other methods by the way JavaScript is read. Function declarations are generally viewed as functions that are moved to the beginning of the code ("hoisted") and may be declared after it is called.
Example:
/*Note the function begins with the keyword 'function'
followed by the name, '()', and '{}'*/
function morningGreeting() {
return 'Good Morning'
}
Function expression is another way of defining a function, differing from a function declaration as it must first be declared prior to being called.
Example:
/*Note the function is identified by
a variable keyword and name*/
followed by being equal to function(){}
const eveningGreeting = function(){
return 'Good Evening'
}
How hoisting works
... hoisting suggests that variable and function declarations are physically moved to the top of your code, but this is not in fact what happens. Instead, the variable and function declarations are put into memory during the compile phase, but stay exactly where you typed them in your code. - MDN web docs
As mentioned in the MDN web docs, we find that all function declarations are stored in memory during the compile phase, prior to being executed. When a function is called that was defined with declarative syntax, it will respond in disregard of where it is written, because it is already stored and ready to be called.
When we write code, we must take in mind how it will be read. Understanding the formalities of how JavaScript is read, granting us greater control of how specific and flexible our code can be. Lack of understanding can result in great difficulties as we debug.
Example: Function declaration
/*Since this is written first, we may have suspected an error.
We will see the function will execute as later defined.*/
console.log((sayHello())
//The following function will be hoisted
function sayHello(){
return 'Hello'
}
//output: 'Hello'
Remember: Only declarations are hoisted
Example: Function Expression
console.log(sayHello())
let sayHello = function(){
return 'Hello'
}
//output: 'undefined'
Rule of Thumb
It is better to use function expressions as they are more specific and thus more controlled. Nevertheless, there is a time and place for declarative functions, and its flexibility should not be overlooked.