Angular Modules
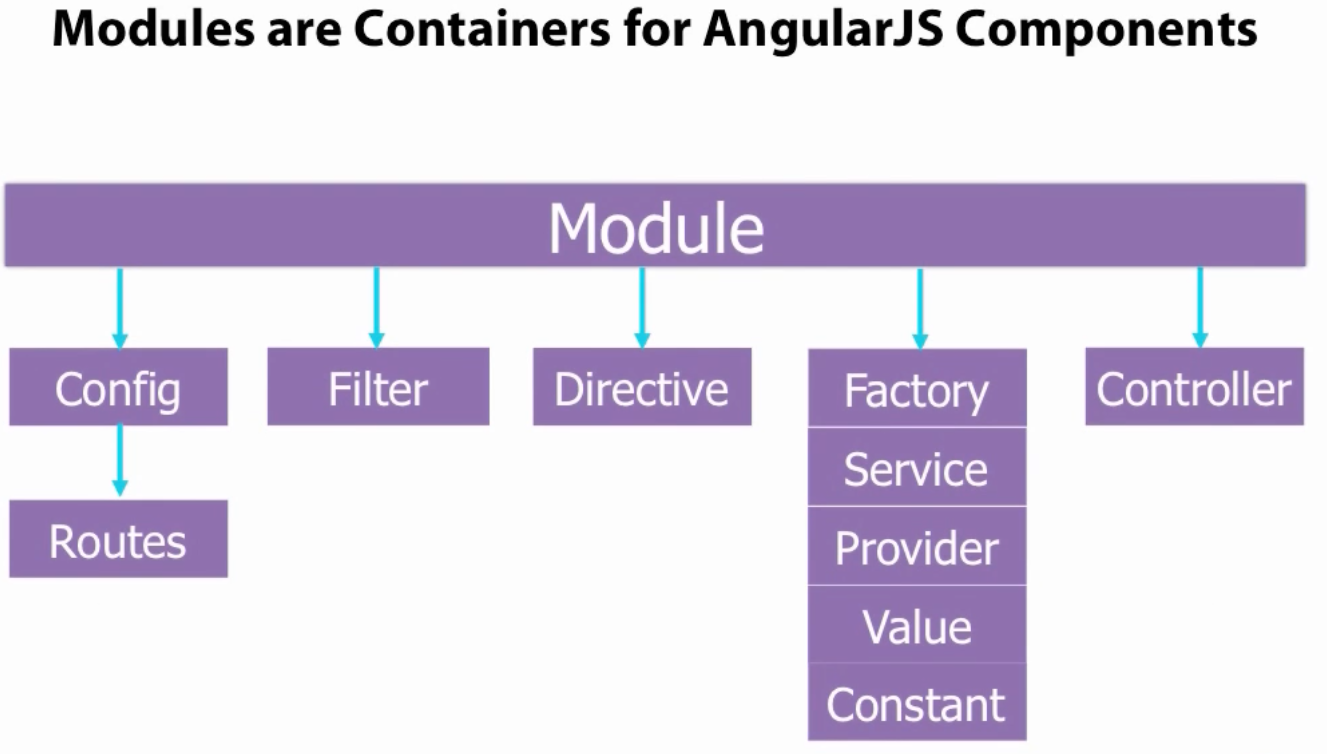
The module is Angular's overall container. It contains all controllers as well as all other AngularJS code. The Angular module is created with the following code in a javascript file:
var app = angular.module("myApp", []);
There are other ways to write this, but we'll stick to this one for now. Let's break it down a bit. The var app
portion is, of course, creating a variable we are calling app
. And app
will be equal to the method angular.module()
. The parameters for this method - "myApp", []
- are the name of the controller (myApp
) and an array of dependancies (currently empty).
Without the []
(Dependency injection array) a new module is not created, rather Angular will look for an already created module of the name specified (myApp
here) and attempt to add its code to it. What does this mean exactly?
var app = angular.module("myApp", []);
The above line creates a module. The line below attempts to add code to the module created above. If the module has not been created an error will be thrown.
var app = angular.module("myApp"); // does NOT create a module
To add angular pieces (controller, directive, etc.) to the module, we would do the following:
var app = angular.module("myApp", []);
app.controller("myCntrl", function($scope) {
// do stuff here
});
Let's break down the line:
We've added a controller (app.controller
) to the myApp
module, we've named the controller myCntrl
, and we've created an anonymous function (function($scope){//do stuff here}
) with the parameter $scope
passed in and some potential code to run when this controller is called.
What is $scope
? We'll cover it the next lesson.
We will always pass in $scope
because it is one of the primary reasons to use Angular. In the future you will pass more things in besides $scope
, but we'll keep it simple for now.
Here's another example one of Angular's building blocks to the module. In this case a directive
var app = angular.module("myApp", []);
app.directive("w3TestDirective", function() {
return {
template : "I was made in a directive constructor!"
};
});
//it already had this one below
app.controller("myCntrl", function($scope) {
// do stuff here
});
You might also have several of something, in this case, several controllers:
var app = angular.module("myApp", []);
app.controller("myCntrl", function($scope) {
// do stuff here
});
app.controller("secondController", function($scope) {
// do different stuff here
});
The module is the core building block or container of Angular and will hold all other Angular building blocks. As a note, a website (app) can utilize more than one module as well.
Let's create 3 different modules.
angular.module(“myapp”, []);
angular.module(“myservices”, []);
angular.module(“mywidgets”, []);
Those modules could all be added to one 'master module' by injecting the above modules into a new module called MainApp
:
angular.module(“MainApp”, [“myapp”, “myservices”, “mywidgets”]);
That's all for now, as you use Angular more the module will become second nature. Good luck...